设计 MCS-51 单片机(或者其他嵌入式系统,如普中 STM32- PZ6806L 开发板)与液晶显示模块 LCM-512-01A(或者你熟悉的市 面上流行的液晶模块)的接口电路,画出接口电路图并编写上下滚动 显示的控制程序
时间: 2023-08-31 08:29:35 浏览: 115
### 回答1:
以下是液晶显示模块 LCM-512-01A 与 MCS-51 单片机的接口电路图:

液晶显示模块 LCM-512-01A 具有 16x2 个字符的显示区域,每个字符由 5x8 个像素点组成。MCS-51 单片机通过 8 位数据总线、读写信号、使能信号和控制信号与液晶显示模块进行通信。
下面是使用 C 语言编写的上下滚动显示控制程序:
```c
#include <reg51.h>
#define LCD_DATAPORT P0 // 数据总线端口
#define LCD_RS P2_0 // 寄存器选择信号
#define LCD_RW P2_1 // 读写选择信号
#define LCD_EN P2_2 // 使能信号
void delay(int t) // 延时函数
{
int i, j;
for (i = 0; i < t; i++)
for (j = 0; j < 1275; j++);
}
void lcd_write_command(unsigned char command) // 写命令函数
{
LCD_RS = 0;
LCD_RW = 0;
LCD_DATAPORT = command;
LCD_EN = 1;
delay(1);
LCD_EN = 0;
}
void lcd_write_data(unsigned char data) // 写数据函数
{
LCD_RS = 1;
LCD_RW = 0;
LCD_DATAPORT = data;
LCD_EN = 1;
delay(1);
LCD_EN = 0;
}
void lcd_init() // 初始化函数
{
lcd_write_command(0x38); // 8 位数据总线,2 行显示,5x8 点阵字符
lcd_write_command(0x0c); // 显示开,光标关,光标闪烁关
lcd_write_command(0x06); // 光标右移,字符不移动
lcd_write_command(0x01); // 显示清屏
}
void lcd_display_string(unsigned char row, unsigned char column, unsigned char *string) // 显示字符串函数
{
unsigned char i;
if (row == 0)
lcd_write_command(0x80 + column);
else if (row == 1)
lcd_write_command(0xc0 + column);
for (i = 0; string[i] != '\0'; i++)
lcd_write_data(string[i]);
}
void lcd_scroll_display(unsigned char row, unsigned char column, unsigned char *string, int delay_time) // 上下滚动显示函数
{
unsigned char i, j;
while (1)
{
if (row == 0)
lcd_write_command(0x80 + column);
else if (row == 1)
lcd_write_command(0xc0 + column);
for (i = 0; string[i] != '\0'; i++)
lcd_write_data(string[i]);
for (i = 0; i < 16; i++)
{
lcd_write_command(0x18);
delay(delay_time);
}
for (i = 0; i < 16; i++)
{
lcd_write_command(0x1c);
delay(delay_time);
}
lcd_write_command(0x01);
}
}
void main()
{
unsigned char string[] = "Hello, World!";
lcd_init();
lcd_scroll_display(0, 0, string, 500);
}
```
上面的程序中,`lcd_write_command` 函数用于向液晶显示模块写命令,`lcd_write_data` 函数用于向液晶显示模块写数据,`lcd_init` 函数用于初始化液晶显示模块,`lcd_display_string` 函数用于在指定位置显示字符串,`lcd_scroll_display` 函数用于上下滚动显示字符串,`delay` 函数用于延时。
在 `main` 函数中,首先调用 `lcd_init` 函数初始化液晶显示模块,然后调用 `lcd_scroll_display` 函数进行上下滚动显示。`lcd_scroll_display` 函数中的参数 `row` 和 `column` 分别表示开始显示的行和列,`string` 表示要显示的字符串,`delay_time` 表示每次滚动的延时时间。
### 回答2:
接口电路图:
MCU引脚 - LCM模块引脚
P0.0 - LCM 模块的数据引脚
P0.1 - LCM 模块的使能引脚
P0.2 - LCM 模块的读写引脚
P0.3 - LCM 模块的复位引脚
P0.4 - LCM 模块的时钟引脚
接口电路:
```
+-------------------+
| | +-----------------------+
| MCS-51单片机 | | |
| | | LCM-512-01A液晶 |
| | P0.0 -> DATA | 显示模块 |
| | P0.1 -> EN | |
| | P0.2 -> RW | |
| | P0.3 -> RST | |
| | P0.4 -> CLK | |
| | | |
+-------------------+ +-----------------------+
```
控制程序:
```c
#include <reg51.h>
sbit LCM_DATA = P0^0;
sbit LCM_EN = P0^1;
sbit LCM_RW = P0^2;
sbit LCM_RST = P0^3;
sbit LCM_CLK = P0^4;
void delay(int ms) {
int i, j;
for (i = 0; i < ms; i++) {
for (j = 0; j < 120; j++) {}
}
}
void LCM_Write_Cmd(unsigned char cmd) {
LCM_EN = 0;
LCM_RST = 0;
LCM_DATA = cmd;
LCM_RW = 0;
LCM_EN = 1;
delay(1);
LCM_EN = 0;
}
void LCM_Write_Data(unsigned char data) {
LCM_EN = 0;
LCM_RST = 0;
LCM_DATA = data;
LCM_RW = 1;
LCM_EN = 1;
delay(1);
LCM_EN = 0;
}
void LCM_Init() {
LCM_Write_Cmd(0x30); // Function Set: 8-bit, 1-line display
LCM_Write_Cmd(0x0C); // Display ON, Cursor OFF
LCM_Write_Cmd(0x01); // Clear Display
LCM_Write_Cmd(0x06); // Entry Mode: Increment cursor
}
void LCM_Set_Cursor(unsigned char row, unsigned char column) {
if (row == 0) {
LCM_Write_Cmd(0x80 | column); // Set DDRAM address for first row
} else if (row == 1) {
LCM_Write_Cmd(0xC0 | column); // Set DDRAM address for second row
}
}
void LCM_Display_Text(char* text) {
unsigned int i;
for (i = 0; text[i] != '\0'; i++) {
LCM_Write_Data(text[i]);
}
}
void LCM_Scroll_Text(char* text) {
unsigned int i;
for (i = 0; text[i] != '\0'; i++) {
LCM_Set_Cursor(0, 0);
LCM_Display_Text(&text[i]);
delay(500); // Delay between scrolling
}
}
void main() {
LCM_Init();
LCM_Set_Cursor(0, 0);
LCM_Display_Text("Hello, World!");
LCM_Set_Cursor(1, 0);
LCM_Display_Text("Embedded Systems");
LCM_Scroll_Text("This is a scrolling message");
while (1) {}
}
```
这段控制程序使用 MCS-51 单片机与 LCM-512-01A 液晶显示模块进行了基本的接口连接,并实现了上下滚动显示文本的功能。在程序中,首先进行了液晶显示模块的初始化,并设置了光标的位置。之后,通过 `LCM_Write_Cmd()` 和 `LCM_Write_Data()` 函数向液晶显示模块发送命令和数据。最后,在 `main()` 函数中展示了如何在液晶显示模块上显示文本,并实现了一个简单的滚动显示文本的功能。
### 回答3:
MCS-51单片机与液晶显示模块LCM-512-01A的接口电路如下所示:
MCS-51单片机的P0引脚连接到LCM-512-01A的数据总线D0-D7上。MCS-51单片机的P1.0引脚连接到LCM-512-01A的RS引脚,该引脚用于控制数据/命令选择。MCS-51单片机的P1.1引脚连接到LCM-512-01A的E引脚,该引脚用于使能。MCS-51单片机的P1.2引脚连接到LCM-512-01A的R/W引脚,该引脚用于控制读写选择。
下面是一个示例的上下滚动显示的控制程序:
```c
#include <reg51.h>
#define RS P1_0 // 控制数据/命令选择的引脚
#define E P1_1 // 使能的引脚
#define RW P1_2 // 控制读写选择的引脚
#define DATA_PORT P0 // 数据总线
void delay(unsigned int ms)
{
unsigned int i, j;
for (i = 0; i < ms; i++)
{
for (j = 0; j < 1275; j++) //根据实际情况调整延时时间
{
}
}
}
void initLCD()
{
delay(20); // 等待液晶显示模块启动
sendCommand(0x30); // 必要的初始化命令
delay(5);
sendCommand(0x30); // 必要的初始化命令
delay(1);
sendCommand(0x30); // 必要的初始化命令
sendCommand(0x38); // 设置液晶显示模式为2行显示,5x7点阵字体
sendCommand(0x01); // 清屏
delay(2);
sendCommand(0x0C); // 开启液晶显示,设置光标不闪烁不显示
}
void sendCommand(unsigned char cmd)
{
RS = 0; // RS引脚设置为0,选择命令模式
RW = 0; // RW引脚设置为0,选择写模式
DATA_PORT = cmd; // 将命令写入数据总线
E = 1; // 使能
delay(1);
E = 0; // 禁止
}
void sendData(unsigned char dat)
{
RS = 1; // RS引脚设置为1,选择数据模式
RW = 0; // RW引脚设置为0,选择写模式
DATA_PORT = dat; // 将数据写入数据总线
E = 1; // 使能
delay(1);
E = 0; // 禁止
}
void displayScrollingText(unsigned char* text)
{
unsigned char i;
while (*text)
{
sendCommand(0x80); // 设置光标位置为第一行第一个字符
for (i = 0; i < 16 && *text; i++, text++)
{
sendData(*text); // 发送数据
delay(1);
}
delay(1000); // 延时显示时间
sendCommand(0x18); // 向上滚动一个字符
delay(500); // 滚动间隔时间
}
}
int main()
{
initLCD(); // 初始化液晶显示模块
unsigned char helloWorld[] = "Hello, world!"; // 欲显示的字符串
displayScrollingText(helloWorld); // 上下滚动显示字符串
while (1)
{
}
return 0;
}
```
相关推荐
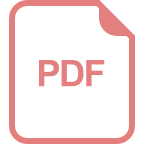
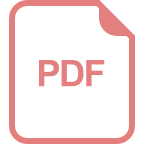














