dijkstra算法 迷宫问题 python
时间: 2023-07-25 18:20:55 浏览: 115
好的,我理解您的问题是关于使用Dijkstra算法解决迷宫问题的Python实现。下面是一个简单的示例代码,希望能对您有所帮助:
```python
import heapq
def dijkstra(maze, start, end):
# 迷宫中可行走的四个方向
directions = [(0, 1), (0, -1), (1, 0), (-1, 0)]
m, n = len(maze), len(maze[0])
# 初始化距离矩阵
distance = [[float('inf')] * n for _ in range(m)]
distance[start[0]][start[1]] = 0
# 初始化堆
heap = [(0, start)]
while heap:
d, node = heapq.heappop(heap)
if node == end:
return d
if distance[node[0]][node[1]] < d:
continue
for direction in directions:
x, y = node[0] + direction[0], node[1] + direction[1]
if 0 <= x < m and 0 <= y < n and maze[x][y] == 0:
new_d = d + 1
if new_d < distance[x][y]:
distance[x][y] = new_d
heapq.heappush(heap, (new_d, (x, y)))
return -1 # 无法到达终点
```
其中,`maze`是迷宫矩阵,0表示可走,1表示墙壁;`start`和`end`分别是起点和终点的坐标,例如`(0, 0)`表示左上角。
这段代码使用了堆优化的Dijkstra算法来求出起点到终点的最短距离。具体实现过程是:
1. 初始化距离矩阵,起点的距离为0,其余点的距离为无穷大;
2. 将起点加入堆中,并以起点为起点,开始向四周扩展;
3. 如果新扩展的点可以到达且距离更短,则更新距离,并将其加入堆中;
4. 重复2-3步骤,直到堆为空或者找到了终点。
最后,如果找到了终点,返回起点到终点的最短距离;否则,说明无法到达终点,返回-1即可。
希望这个示例代码对您有所帮助!
阅读全文
相关推荐
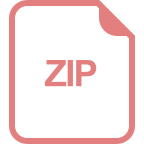
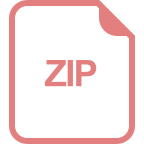

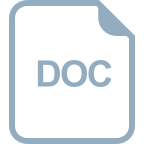
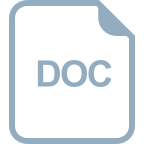


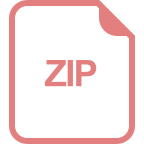
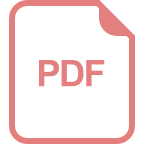
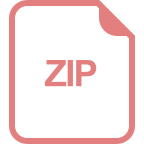
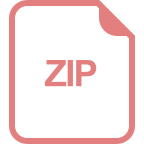
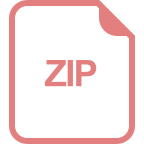
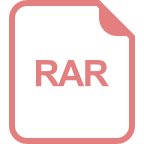
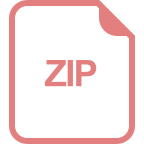
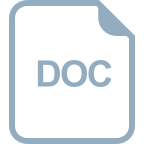
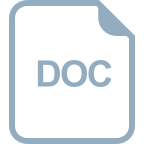
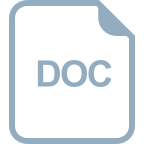
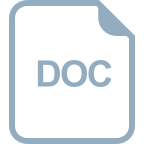