写几个linq的代码示范
时间: 2023-12-01 13:05:01 浏览: 64
1. 查询数组中的偶数:
```
int[] numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9 };
var evenNumbers = numbers.Where(n => n % 2 == 0);
```
2. 查询字符串列表中长度大于等于5的字符串:
```
List<string> fruits = new List<string> { "apple", "banana", "orange", "grape", "kiwi" };
var longFruits = fruits.Where(f => f.Length >= 5);
```
3. 查询学生列表中年龄小于20岁的学生:
```
public class Student
{
public string Name { get; set; }
public int Age { get; set; }
}
List<Student> students = new List<Student>
{
new Student { Name = "Tom", Age = 18 },
new Student { Name = "Mary", Age = 21 },
new Student { Name = "John", Age = 19 },
new Student { Name = "Alice", Age = 22 }
};
var youngStudents = students.Where(s => s.Age < 20);
```
4. 查询员工列表中薪水大于5000的员工姓名:
```
public class Employee
{
public string Name { get; set; }
public int Salary { get; set; }
}
List<Employee> employees = new List<Employee>
{
new Employee { Name = "Tom", Salary = 6000 },
new Employee { Name = "Mary", Salary = 4500 },
new Employee { Name = "John", Salary = 5500 },
new Employee { Name = "Alice", Salary = 5000 }
};
var highSalaryEmployees = employees.Where(e => e.Salary > 5000).Select(e => e.Name);
```
阅读全文
相关推荐
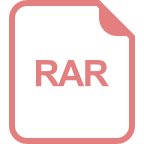
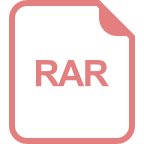
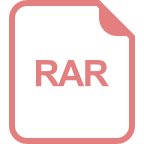
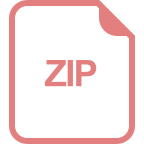
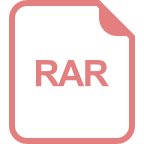
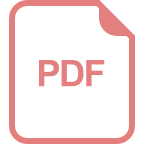
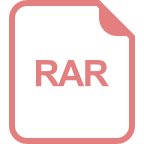
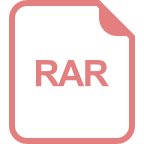
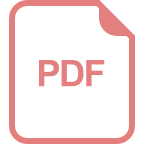
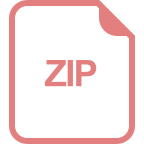
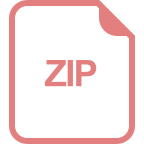
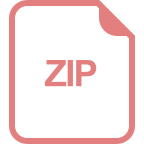
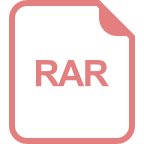
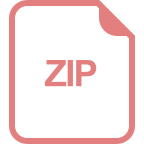