C++的deque所需内存太大了,如果我仅需一个容器,能支持快速头尾插入和随机访问,怎么实现内存较小?
时间: 2023-02-07 15:20:01 浏览: 169
在 C 语言中,你可以使用链表来实现一个容器,它可以支持快速头尾插入和随机访问,并且所需的内存也相对较小。你可以使用结构体来定义链表中的每个节点,其中包含一个存储数据的字段和两个指针字段,用于指向前一个节点和后一个节点。通过移动这些指针,你就可以在链表的头部或尾部插入新节点,也可以在链表中随机访问任意节点。
例如,下面是一个使用链表实现的简单堆栈,它支持 push 和 pop 操作:
```
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node* next;
};
struct stack {
struct node* head;
};
void push(struct stack* s, int data) {
struct node* new_node = (struct node*) malloc(sizeof(struct node));
new_node->data = data;
new_node->next = s->head;
s->head = new_node;
}
int pop(struct stack* s) {
if (s->head == NULL) {
printf("Error: stack is empty\n");
exit(1);
}
int data = s->head->data;
struct node* temp = s->head;
s->head = s->head->next;
free(temp);
return data;
}
int main() {
struct stack s;
s.head = NULL;
push(&s, 1);
push(&s, 2);
push(&s, 3);
printf("%d\n", pop(&s));
printf("%d\n", pop(&s));
printf("%d\n", pop(&s));
return 0;
}
```
链表的一个缺点是随机访问时间复杂度较高,因为你需要从
相关推荐
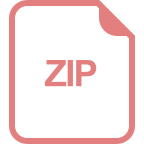














