android语音机器人代码,Android studio开发小型对话机器人app(实例代码)
时间: 2024-02-24 12:58:46 浏览: 23
以下是一个简单的 Android 语音机器人代码示例,使用 Android Studio 开发:
1. 首先,在 Android Studio 中创建一个新项目。
2. 在项目的 MainActivity.java 文件中,添加以下代码:
```java
import android.speech.tts.TextToSpeech;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import java.util.Locale;
public class MainActivity extends AppCompatActivity implements TextToSpeech.OnInitListener {
private TextToSpeech tts;
private EditText editText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tts = new TextToSpeech(this, this);
editText = (EditText) findViewById(R.id.editText);
Button button = (Button) findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String text = editText.getText().toString();
speak(text);
}
});
}
@Override
public void onInit(int status) {
if (status == TextToSpeech.SUCCESS) {
int result = tts.setLanguage(Locale.US);
if (result == TextToSpeech.LANG_MISSING_DATA || result == TextToSpeech.LANG_NOT_SUPPORTED) {
// Language data is missing or the language is not supported.
}
} else {
// Initialization failed.
}
}
private void speak(String text) {
tts.speak(text, TextToSpeech.QUEUE_FLUSH, null, null);
}
@Override
protected void onDestroy() {
if (tts != null) {
tts.stop();
tts.shutdown();
}
super.onDestroy();
}
}
```
3. 在项目的 activity_main.xml 文件中,添加以下代码:
```xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:hint="Enter text to speak" />
<Button
android:id="@+id/button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/editText"
android:layout_margin="16dp"
android:text="Speak" />
</RelativeLayout>
```
4. 运行应用程序,并在文本框中输入一些文本,然后点击“Speak”按钮,语音机器人会使用 TextToSpeech API 朗读出输入的文本。
这只是一个简单的示例,你可以根据自己的需求进行修改和扩展。
相关推荐
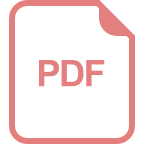
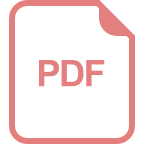
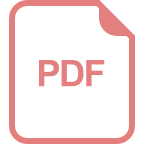














