数据结构c语言编程在舞会上,男、女各自排成一队。舞会开始时,依次从男队和女队各出一人配成舞伴。如果两队初始人数不等,则较长的那一对中未配对者等待下一轮舞曲,舞曲结束后,按照先配对的先分开进入各自的队列。假设初始男、女人数及舞会的轮数由参数给出,模拟上述舞伴配对问题。
时间: 2024-11-27 08:13:54 浏览: 4
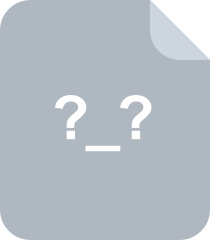
C语言高级编程基础数据结构(第一部分:绪论)
在C语言中,你可以使用数组或链表来表示男队和女队的人数,并通过循环或递归来模拟这种舞蹈配对的过程。以下是一个简单的伪代码描述:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int gender; // 0 for men, 1 for women
int index; // Index of the person in their respective queue
} Person;
void dance(int* men_count, int* women_count, int rounds) {
Person* men = (Person*)malloc(sizeof(Person) * (*men_count));
Person* women = (Person*)malloc(sizeof(Person) * (*women_count));
// Initialize the queues
for (int i = 0; i < *men_count; i++) {
men[i].gender = 0;
men[i].index = i;
}
for (int j = 0; j < *women_count; j++) {
women[j].gender = 1;
women[j].index = j;
}
for (int round = 0; round < rounds; round++) {
if (*men_count > *women_count) {
Person next_woman = women[(*women_count)-1];
women[*women_count - 1] = women[0]; // Move last woman to front
free(&women[0]); // Remove last one from list
*women_count -= 1;
} else if (*women_count > *men_count) {
Person next_man = men[(*men_count)-1];
men[*men_count - 1] = men[0]; // Move last man to front
free(&men[0]);
*men_count -= 1;
} else { // Both have same count
Person next_pair = men[(*men_count)-1];
men[*men_count - 1] = men[0];
free(&men[0]);
next_pair.index++; // Increment the index since they're paired
men[*men_count] = next_pair; // Add back to queue
next_pair = women[(*women_count)-1];
women[*women_count - 1] = women[0];
free(&women[0]);
next_pair.index++; // Same for women
women[*women_count] = next_pair;
}
}
// Print remaining partners (if any)
printf("Remaining after %d rounds:\n", rounds);
for (int i = 0; i < *men_count; i++) {
printf("Man %d: (%d,%d)\n", i+1, men[i].gender, men[i].index);
}
for (int j = 0; j < *women_count; j++) {
printf("Woman %d: (%d,%d)\n", j+1, women[j].gender, women[j].index);
}
}
// Example usage
int main() {
int men = 5, women = 3, rounds = 4;
dance(&men, &women, rounds);
return 0;
}
```
阅读全文
相关推荐
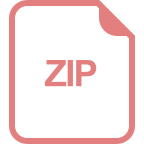















