uec++中的接口如何实现不同类的通信
时间: 2024-06-11 19:11:03 浏览: 167
在UEC中,实现不同类的通信可以通过接口实现。具体步骤如下:
1. 定义接口:定义一个接口,包含需要实现的方法。
2. 实现接口:实现这个接口的类需要实现接口中的方法,这样就可以保证这些类都有相同的方法。
3. 使用接口:在其他类中使用这个接口,这样就可以调用实现了这个接口的类中的方法。
例如,我们可以定义一个名为“printable”的接口,其中包含一个“print()”方法。然后我们可以定义两个类,“Person”和“Animal”,分别实现这个接口。在其他类中,我们可以使用“printable”接口来调用这两个类的“print()”方法。
这样,不同类之间就可以通过接口实现通信。接口提供了一种封装和抽象的方式,使得实现接口的类可以被更加灵活地使用。
相关问题
能举一个uec++中通过接口实现类与类之间通信的例子吗
假设有两个类 A 和 B,它们需要通过接口实现通信。可以定义一个接口 ICommunication,包含一个方法 communicate(),然后让 A 和 B 分别实现这个接口。
示例代码如下:
```java
public interface ICommunication {
void communicate();
}
public class A implements ICommunication {
private B b;
public A(B b) {
this.b = b;
}
@Override
public void communicate() {
// 调用 B 的方法
b.process();
}
}
public class B implements ICommunication {
private A a;
public B(A a) {
this.a = a;
}
@Override
public void communicate() {
// 调用 A 的方法
a.process();
}
public void process() {
// 处理逻辑
}
}
```
在这个例子中,A 和 B 通过接口 ICommunication 实现了通信。A 和 B 分别持有对方的实例作为成员变量,这样就可以在 communicate() 方法中调用对方的方法。这种方式可以帮助我们实现松耦合的代码设计,提高代码的可维护性和可扩展性。
UEC++中 ,通过接口实现类与类之间通信的例子
下面是一个通过接口实现类与类之间通信的例子:
假设有一个接口 `ICommunication`,定义了一个方法 `sendMessage(String message)`。现在有两个类 `Sender` 和 `Receiver`,它们需要通过该接口进行通信。
Sender 类中的代码如下:
```java
public class Sender {
private ICommunication communication;
public Sender(ICommunication communication) {
this.communication = communication;
}
public void sendMessage(String message) {
communication.sendMessage(message);
}
}
```
Receiver 类中的代码如下:
```java
public class Receiver implements ICommunication {
public void receiveMessage(String message) {
System.out.println("Received message: " + message);
}
@Override
public void sendMessage(String message) {
// This method is not used in Receiver class
}
}
```
在上面的代码中,Sender 类的构造函数接收一个 `ICommunication` 类型的参数,并将其保存到 `communication` 成员变量中。然后,Sender 类中的 `sendMessage` 方法调用了 `communication` 变量的 `sendMessage` 方法,从而向 Receiver 类发送消息。
Receiver 类实现了 `ICommunication` 接口,并实现了 `receiveMessage` 方法。在这个方法中,Receiver 类接收到了发送者发送的消息,并打印出来。
现在,我们可以在主函数中创建 Sender 和 Receiver 对象,并让它们进行通信:
```java
public static void main(String[] args) {
Receiver receiver = new Receiver();
Sender sender = new Sender(receiver);
sender.sendMessage("Hello, world!");
}
```
在上面的代码中,我们首先创建了一个 Receiver 对象。然后,我们创建了一个 Sender 对象,并将 Receiver 对象传递给了它。最后,我们调用了 Sender 对象的 `sendMessage` 方法,从而向 Receiver 对象发送了一条消息。
通过这个例子,我们可以看到,通过接口实现类与类之间通信是非常方便和灵活的。在这个例子中,我们只需要定义一个接口,并让 Sender 和 Receiver 类实现该接口,就可以实现它们之间的通信。这种方式可以有效地降低类之间的耦合度,从而使代码更加可维护和可扩展。
阅读全文
相关推荐
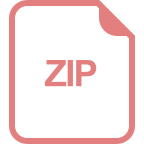
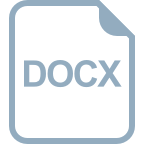
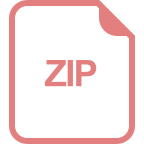

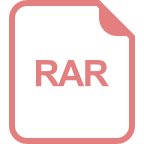
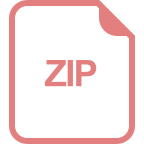
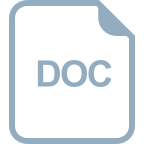
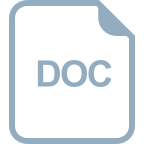
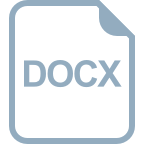
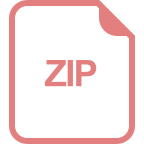
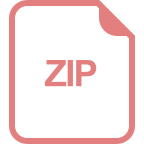
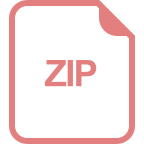
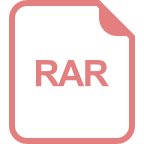
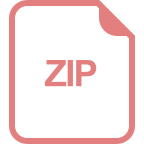
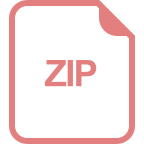