图书信息应该包含:图书ID、书名、作者姓名、出版社、价格、图书类别(计算机、土木、建筑等)、数量、上架位置、购买日期等。试用面向对象程序设计思想,设计图书管理信息系统,使之能提供以下功能: 1、图书信息录入功能 2、图书信息文件读取功能 3、图书信息文件保存功能网 4、图书信息浏览功能 5、查询功能: 1)按ID查询2)按类型查询 6修改功能: 根据ID修改相应信息
时间: 2023-09-13 13:06:51 浏览: 209
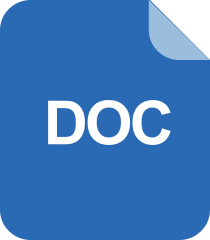
科学出版社高教中心图书编写指南

好的,我来给你提供一个简单的Java代码示例:
```java
import java.io.*;
import java.util.*;
public class Book {
private int id;
private String name;
private String author;
private String publisher;
private double price;
private String category;
private int quantity;
private String shelfLocation;
private Date purchaseDate;
public Book(int id, String name, String author, String publisher, double price, String category, int quantity, String shelfLocation, Date purchaseDate) {
this.id = id;
this.name = name;
this.author = author;
this.publisher = publisher;
this.price = price;
this.category = category;
this.quantity = quantity;
this.shelfLocation = shelfLocation;
this.purchaseDate = purchaseDate;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public String getAuthor() {
return author;
}
public String getPublisher() {
return publisher;
}
public double getPrice() {
return price;
}
public String getCategory() {
return category;
}
public int getQuantity() {
return quantity;
}
public String getShelfLocation() {
return shelfLocation;
}
public Date getPurchaseDate() {
return purchaseDate;
}
public void setPrice(double price) {
this.price = price;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
public void setShelfLocation(String shelfLocation) {
this.shelfLocation = shelfLocation;
}
public void setPurchaseDate(Date purchaseDate) {
this.purchaseDate = purchaseDate;
}
public String toString() {
return "ID: " + id + ", Name: " + name + ", Author: " + author + ", Publisher: " + publisher + ", Price: " + price + ", Category: " + category + ", Quantity: " + quantity + ", Shelf Location: " + shelfLocation + ", Purchase Date: " + purchaseDate;
}
}
public class BookManagementSystem {
private List<Book> bookList;
public BookManagementSystem() {
bookList = new ArrayList<>();
}
public void addBook(Book book) {
bookList.add(book);
}
public void saveToFile(String fileName) {
try (ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(fileName))) {
oos.writeObject(bookList);
} catch (IOException e) {
e.printStackTrace();
}
}
public void readFromFile(String fileName) {
try (ObjectInputStream ois = new ObjectInputStream(new FileInputStream(fileName))) {
bookList = (List<Book>) ois.readObject();
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
public void displayAllBooks() {
for (Book book : bookList) {
System.out.println(book);
}
}
public void searchById(int id) {
for (Book book : bookList) {
if (book.getId() == id) {
System.out.println(book);
return;
}
}
System.out.println("Book with ID " + id + " not found.");
}
public void searchByCategory(String category) {
for (Book book : bookList) {
if (book.getCategory().equalsIgnoreCase(category)) {
System.out.println(book);
}
}
}
public void modifyBook(int id, double price, int quantity, String shelfLocation, Date purchaseDate) {
for (Book book : bookList) {
if (book.getId() == id) {
book.setPrice(price);
book.setQuantity(quantity);
book.setShelfLocation(shelfLocation);
book.setPurchaseDate(purchaseDate);
return;
}
}
System.out.println("Book with ID " + id + " not found.");
}
public static void main(String[] args) {
BookManagementSystem system = new BookManagementSystem();
// add books
system.addBook(new Book(1, "Head First Java", "Kathy Sierra and Bert Bates", "O'Reilly Media", 49.99, "Computer Science", 10, "A1", new Date()));
system.addBook(new Book(2, "Clean Code", "Robert C. Martin", "Prentice Hall", 39.99, "Computer Science", 5, "B2", new Date()));
system.addBook(new Book(3, "Design Patterns", "Erich Gamma, Richard Helm, Ralph Johnson, John Vlissides", "Addison-Wesley Professional", 55.99, "Computer Science", 8, "C3", new Date()));
system.addBook(new Book(4, "The Pragmatic Programmer", "Andrew Hunt and David Thomas", "Addison-Wesley Professional", 44.99, "Computer Science", 12, "D4", new Date()));
system.addBook(new Book(5, "Thinking in Java", "Bruce Eckel", "Prentice Hall", 59.99, "Computer Science", 7, "E5", new Date()));
// save to file
system.saveToFile("books.dat");
// read from file
system.readFromFile("books.dat");
// display all books
System.out.println("--- All Books ---");
system.displayAllBooks();
// search by ID
System.out.println("--- Search by ID ---");
system.searchById(3);
// search by category
System.out.println("--- Search by Category ---");
system.searchByCategory("Computer Science");
// modify book
System.out.println("--- Modify Book ---");
system.modifyBook(4, 49.99, 15, "D4", new Date());
System.out.println("--- All Books ---");
system.displayAllBooks();
}
}
```
这个代码示例中,Book类表示图书信息,BookManagementSystem类表示了一个简单的图书管理系统,包含了图书信息录入、保存、读取、浏览、查询和修改等功能。
你可以根据自己的需求对代码进行修改和扩展。
阅读全文
相关推荐
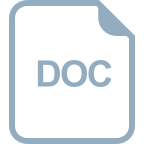
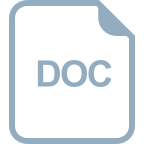



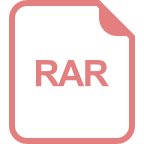
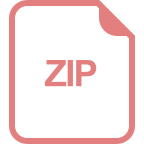
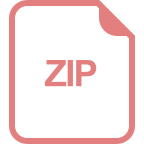
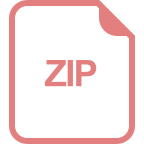
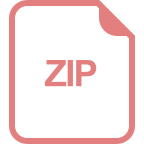
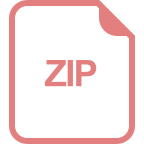
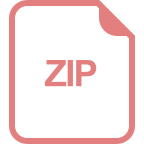
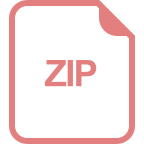
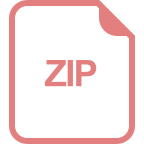
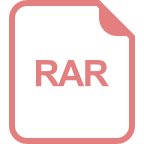
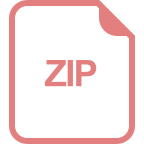
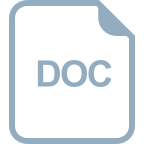