如何在C#中使用SHA-256对密码进行哈希?
时间: 2024-12-18 15:34:07 浏览: 24
在C#中,你可以使用`System.Security.Cryptography.SHA256Managed`类来对密码进行SHA-256哈希。以下是一个简单的示例:
```csharp
using System;
using System.Security.Cryptography;
public class SHA256HashExample
{
public static string HashPassword(string password)
{
// 创建SHA256算法实例
using (SHA256 sha256Hash = SHA256.Create())
{
// 对密码进行加密
byte[] bytes = sha256Hash.ComputeHash(Encoding.UTF8.GetBytes(password));
// 转换成十六进制字符串
StringBuilder builder = new StringBuilder();
foreach (byte b in bytes)
builder.Append(b.ToString("x2"));
return builder.ToString();
}
}
public static void Main()
{
string password = "examplePassword";
string hashedPassword = HashPassword(password);
Console.WriteLine($"Original password: {password}");
Console.WriteLine($"Hashed password (SHA-256): {hashedPassword}");
}
}
```
在这个示例中,我们首先导入所需的命名空间,然后定义一个`HashPassword`方法,该方法接收一个字符串参数(密码),对其进行UTF-8编码并计算SHA-256哈希。最后,我们将哈希结果转换为十六进制字符串返回。
运行`Main`方法,你会看到原始密码及其对应的SHA-256哈希值。
阅读全文
相关推荐










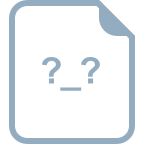





