用c++程序完善自定义字符串类 mystring,函数包括构造函数、复制构造西数、析构的数,并重载“[]”、〞=”、“+”(连接),“ <”、“ >”、“==”(比较)。
时间: 2023-11-05 14:28:51 浏览: 90
好的,我会尽力回答你的问题。首先,我需要明确你的问题:你想让我提供一个示例C++程序,用于完善自定义字符串类mystring,其中包括构造函数、复制构造函数、析构函数,并重载[]、=、+、<、>、==等操作符。如果我理解得有误,请及时纠正我。
以下是一个示例程序,供你参考:
```c++
#include <iostream>
#include <cstring>
class mystring {
public:
mystring(); // 默认构造函数
mystring(const char *str); // 构造函数
mystring(const mystring &); // 复制构造函数
~mystring(); // 析构函数
char& operator[](int index); // 重载[]操作符
mystring& operator=(const mystring &); // 重载=操作符
mystring operator+(const mystring &) const; // 重载+操作符
bool operator<(const mystring &) const; // 重载<操作符
bool operator>(const mystring &) const; // 重载>操作符
bool operator==(const mystring &) const; // 重载==操作符
const char* c_str() const { return str_; } // 返回字符串
private:
char *str_; // 字符串
};
mystring::mystring() : str_(new char[1]) {
*str_ = '\0'; // 默认构造函数,创建一个空字符串
}
mystring::mystring(const char *str) : str_(new char[strlen(str) + 1]) {
strcpy(str_, str); // 构造函数,根据指定字符串创建一个新字符串
}
mystring::mystring(const mystring &rhs) : str_(new char[rhs.size() + 1]) {
strcpy(str_, rhs.str_); // 复制构造函数,根据已有字符串创建一个新字符串
}
mystring::~mystring() {
delete[] str_; // 析构函数,释放内存
}
char& mystring::operator[](int index) {
return str_[index]; // 返回指定下标的字符
}
mystring& mystring::operator=(const mystring &rhs) {
if (this != &rhs) { // 避免自我赋值
delete[] str_; // 释放原有内存
str_ = new char[rhs.size() + 1]; // 分配新的内存
strcpy(str_, rhs.str_); // 复制字符串
}
return *this; // 返回当前对象的引用
}
mystring mystring::operator+(const mystring &rhs) const {
mystring tmp;
delete[] tmp.str_;
tmp.str_ = new char[size() + rhs.size() + 1];
strcpy(tmp.str_, str_);
strcat(tmp.str_, rhs.str_);
return tmp; // 返回连接后的新字符串
}
bool mystring::operator<(const mystring &rhs) const {
return strcmp(str_, rhs.str_) < 0; // 按字典序比较两个字符串
}
bool mystring::operator>(const mystring &rhs) const {
return strcmp(str_, rhs.str_) > 0; // 按字典序比较两个字符串
}
bool mystring::operator==(const mystring &rhs) const {
return strcmp(str_, rhs.str_) == 0; // 判断两个字符串是否相等
}
int main() {
mystring s1("hello");
mystring s2("world");
mystring s3;
s3 = s1 + s2;
std::cout << s3.c_str() << std::endl;
if (s1 < s2) {
std::cout << "s1 < s2" << std::endl;
} else {
std::cout << "s1 >= s2" << std::endl;
}
if (s1 == s2) {
std::cout << "s1 == s2" << std::endl;
} else {
std::cout << "s1 != s2" << std::endl;
}
return 0;
}
```
这个示例程序实现了一个简单的mystring类,包括默认构造函数、构造函数、复制构造函数、析构函数、[]、=、+、<、>、==等操作符的重载。当你使用这个类时,你可以像操作普通字符串一样来操作mystring对象。
当然,这只是一个示例程序,并不是一个完整的实现。如果你想使用这个类,你需要进一步完善它,例如添加更多的操作符重载、处理异常、优化性能等。
阅读全文
相关推荐
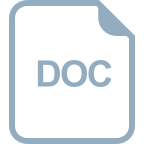














