python ftp远程创建层级目录
时间: 2023-08-30 08:04:12 浏览: 85
使用Python中的ftplib模块,可以通过FTP协议在远程服务器上创建层级目录。具体的操作步骤如下:
1. 连接FTP服务器:使用ftplib模块中的FTP类,创建一个FTP连接对象,并使用connect方法连接到FTP服务器。例如:
```
from ftplib import FTP
ftp = FTP()
ftp.connect('ftp.example.com', 21)
ftp.login('username', 'password')
```
2. 创建层级目录:使用ftp.mkd方法创建目录,该方法接受一个参数,表示要创建的目录名称。例如,如果要在FTP服务器上创建一个名为“dir1/dir2”的目录,可以使用以下代码:
```
ftp.mkd('dir1')
ftp.cwd('dir1')
ftp.mkd('dir2')
```
上述代码先创建了一个名为“dir1”的目录,然后使用cwd方法进入该目录,并在该目录下创建了一个名为“dir2”的目录。需要注意的是,如果要创建的目录已经存在,ftp.mkd方法会返回一个“目录已存在”的错误。
3. 关闭FTP连接:使用ftp.quit方法关闭FTP连接。例如:
```
ftp.quit()
```
完成上述步骤后,就可以在FTP服务器上创建层级目录了。需要注意的是,如果要创建多层目录,需要先创建上层目录,再逐级创建下层目录。
相关问题
python创建FTP远程目录
可以使用ftplib库来创建FTP远程目录。以下是一个示例代码:
```python
import ftplib
# 连接FTP服务器
ftp = ftplib.FTP("ftp.example.com")
ftp.login("username", "password")
# 创建目录
directory = "/remote/directory/path"
ftp.mkd(directory)
# 关闭FTP连接
ftp.quit()
```
请将上述代码中的"ftp.example.com"、"username"、"password"、"/remote/directory/path"替换为你的FTP服务器地址、登录账号、登录密码、以及需要创建的远程目录路径。
python ftp创建目录
Python中使用ftplib模块可以通过ftp.mkd(pathname)方法在FTP服务器上创建目录。具体步骤如下:
1. 连接FTP服务器并登录;
2. 使用ftp.cwd(pathname)方法切换到需要创建目录的父目录;
3. 使用ftp.mkd(pathname)方法创建目录;
4. 关闭FTP连接。
示例代码如下:
```
from ftplib import FTP
ftp = FTP()
ftp.connect('ftp.server.com', 21)
ftp.login('username', 'password')
# 切换到需要创建目录的父目录
ftp.cwd('/home/username/')
# 创建目录
ftp.mkd('new_directory')
# 关闭FTP连接
ftp.quit()
```
相关推荐
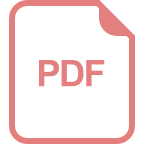












