用C++模拟实现动态分区存储管理,要求: 分区分配算法至少实现首次适应算法、最佳适应算法和最坏适应算法中的至少一种。熟悉并掌握各种算法的空闲区组织方式。 分区的初始化——可以由用户输入初始分区的大小。(初始化后只有一个空闲分区,起始地址为0,大小是用户输入的大小) 分区的动态分配过程:由用户输入作业号和作业的大小,实现分区过程。 分区的回收:用户输入作业号,实现分区回收,同时,分区的合并要体现出来。(注意:不存在的作业号要给出错误提示!) 分区的显示:任何时刻,可以查看当前内存的情况(起始地址是什么,大小多大的分区时空闲的,或者占用的,能够显示出来) 要求考虑:(1)内存空间不足的情况,要有相应的显示; (2)作业不能同名,但是删除后可以再用这个名字; (3)作业空间回收是输入作业名,回收相应的空间,如果这个作业名不存在,也要有相应的提示。
时间: 2024-02-13 12:04:01 浏览: 105
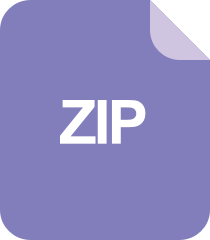
基于 c++实现 内存管理 - 动态分区分配方式模拟 代码详解教程

以下是一个用C++实现动态分区存储管理的示例代码,实现了首次适应算法、最佳适应算法和最坏适应算法,以及分区的初始化、动态分配、回收和显示功能。
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
// 分区结构体
struct Partition {
int start_addr; // 起始地址
int size; // 大小
bool free; // 是否空闲
string job; // 作业名
};
// 分区表
vector<Partition> partitions;
// 初始化分区
void init_partitions(int size) {
partitions.clear();
Partition p = {0, size, true, ""};
partitions.push_back(p);
}
// 首次适应算法
int first_fit(int size) {
for (int i = 0; i < partitions.size(); i++) {
if (partitions[i].free && partitions[i].size >= size) {
return i;
}
}
return -1;
}
// 最佳适应算法
int best_fit(int size) {
int best_idx = -1;
int min_size = INT_MAX;
for (int i = 0; i < partitions.size(); i++) {
if (partitions[i].free && partitions[i].size >= size && partitions[i].size < min_size) {
best_idx = i;
min_size = partitions[i].size;
}
}
return best_idx;
}
// 最坏适应算法
int worst_fit(int size) {
int worst_idx = -1;
int max_size = -1;
for (int i = 0; i < partitions.size(); i++) {
if (partitions[i].free && partitions[i].size >= size && partitions[i].size > max_size) {
worst_idx = i;
max_size = partitions[i].size;
}
}
return worst_idx;
}
// 分配空间
void allocate(string job, int size, int algorithm) {
int idx;
switch (algorithm) {
case 1:
idx = first_fit(size);
break;
case 2:
idx = best_fit(size);
break;
case 3:
idx = worst_fit(size);
break;
default:
cout << "Invalid algorithm" << endl;
return;
}
if (idx == -1) {
cout << "Memory allocation failed for job " << job << endl;
return;
}
partitions[idx].free = false;
partitions[idx].job = job;
// 如果分区大小大于作业大小,需要将剩余空间插入到分区表中
if (partitions[idx].size > size) {
Partition p = {partitions[idx].start_addr + size, partitions[idx].size - size, true, ""};
partitions.insert(partitions.begin() + idx + 1, p);
partitions[idx].size = size;
}
cout << "Job " << job << " allocated " << size << " bytes starting from memory location " << partitions[idx].start_addr << endl;
}
// 回收空间
void deallocate(string job) {
bool found = false;
for (int i = 0; i < partitions.size(); i++) {
if (partitions[i].job == job) {
partitions[i].free = true;
partitions[i].job = "";
// 如果前后分区都是空闲的,需要将它们合并
if (i > 0 && partitions[i - 1].free) {
partitions[i - 1].size += partitions[i].size;
partitions.erase(partitions.begin() + i);
i--;
}
if (i < partitions.size() - 1 && partitions[i + 1].free) {
partitions[i].size += partitions[i + 1].size;
partitions.erase(partitions.begin() + i + 1);
}
found = true;
cout << "Job " << job << " deallocated from memory starting from location " << partitions[i].start_addr << endl;
break;
}
}
if (!found) {
cout << "Job " << job << " not found" << endl;
}
}
// 显示分区情况
void show_partitions() {
cout << "========================================" << endl;
cout << "Start address\tSize\tFree\tJob" << endl;
cout << "========================================" << endl;
for (int i = 0; i < partitions.size(); i++) {
cout << partitions[i].start_addr << "\t\t" << partitions[i].size << "\t" << partitions[i].free << "\t" << partitions[i].job << endl;
}
cout << "========================================" << endl;
}
int main() {
int size;
cout << "Enter initial size of partition: ";
cin >> size;
init_partitions(size);
while (true) {
int choice, algorithm;
string job;
int size;
cout << "1. Allocate memory" << endl;
cout << "2. Deallocate memory" << endl;
cout << "3. Show partitions" << endl;
cout << "4. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
cout << "Enter job name: ";
cin >> job;
cout << "Enter job size: ";
cin >> size;
cout << "Enter allocation algorithm (1 for first fit, 2 for best fit, 3 for worst fit): ";
cin >> algorithm;
allocate(job, size, algorithm);
break;
case 2:
cout << "Enter job name: ";
cin >> job;
deallocate(job);
break;
case 3:
show_partitions();
break;
case 4:
return 0;
default:
cout << "Invalid choice" << endl;
}
}
return 0;
}
```
这段代码实现了一个简单的动态分区存储管理。`Partition` 结构体表示一个分区的信息,包括起始地址、大小、是否空闲和作业名。`partitions` 向量表示所有分区的分区表。
`init_partitions` 函数初始化分区表,只有一个空闲分区,起始地址为0,大小是用户输入的大小。`first_fit`、`best_fit` 和 `worst_fit` 函数分别实现了首次适应算法、最佳适应算法和最坏适应算法,返回满足要求的分区的下标。
`allocate` 函数根据用户输入的作业名和大小以及分配算法分配空间。根据算法在分区表中找到满足要求的空闲分区,将其标记为占用,并更新作业名。如果分区大小大于作业大小,则需要将剩余空间插入到分区表中。
`deallocate` 函数根据用户输入的作业名回收空间。在分区表中找到对应的分区,将其标记为空闲,并更新作业名。如果前后分区都是空闲的,需要将它们合并。
`show_partitions` 函数打印当前分区情况。在控制台输出分区表的每一行。
在 `main` 函数中,我们首先根据用户输入的大小初始化分区表。然后进入一个循环,根据用户的选择进行分配、回收、显示或退出操作。
阅读全文
相关推荐
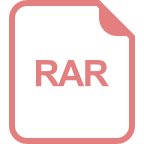
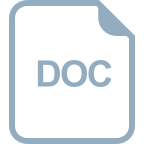
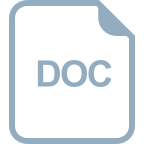
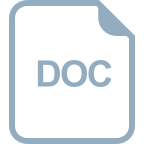
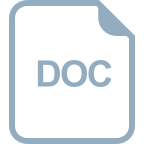

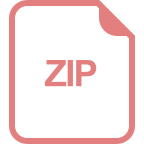
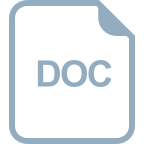
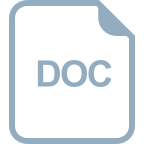
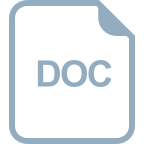
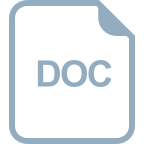
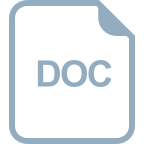


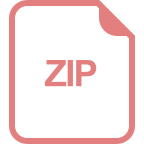
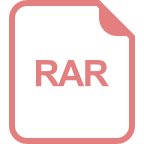
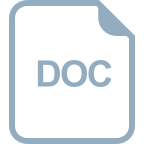