3D-LMNet代码怎么实现
时间: 2023-12-03 16:46:18 浏览: 146
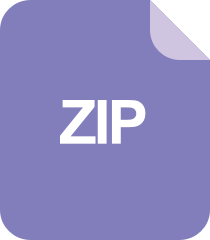
3D-LMNet存储库:用于从单个图像重建准确和多样化的3D点云的潜在嵌入匹配[BMVC2018]_Python_C++.zip
3D-LMNet是一种3D图像分割网络,其结构类似于2D图像分割的U-Net网络。下面是一个示例代码,仅供参考:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class DoubleConv3D(nn.Module):
def __init__(self, in_channels, out_channels):
super().__init__()
self.conv = nn.Sequential(
nn.Conv3d(in_channels, out_channels, kernel_size=3, padding=1),
nn.BatchNorm3d(out_channels),
nn.ReLU(inplace=True),
nn.Conv3d(out_channels, out_channels, kernel_size=3, padding=1),
nn.BatchNorm3d(out_channels),
nn.ReLU(inplace=True)
)
def forward(self, x):
return self.conv(x)
class Down3D(nn.Module):
def __init__(self, in_channels, out_channels):
super().__init__()
self.mpconv = nn.Sequential(
nn.MaxPool3d(2),
DoubleConv3D(in_channels, out_channels)
)
def forward(self, x):
return self.mpconv(x)
class Up3D(nn.Module):
def __init__(self, in_channels, out_channels, bilinear=True):
super().__init__()
# 使用双线性插值进行上采样
if bilinear:
self.up = nn.Upsample(scale_factor=2, mode='bilinear', align_corners=True)
else:
self.up = nn.ConvTranspose3d(in_channels // 2, in_channels // 2, kernel_size=2, stride=2)
self.conv = DoubleConv3D(in_channels, out_channels)
def forward(self, x1, x2):
x1 = self.up(x1)
# x1是上采样后的特征图,x2是从编码器中传递下来的特征图
diffZ = x1.size()[2] - x2.size()[2]
diffY = x1.size()[3] - x2.size()[3]
diffX = x1.size()[4] - x2.size()[4]
x2 = F.pad(x2, (diffX // 2, diffX - diffX // 2,
diffY // 2, diffY - diffY // 2,
diffZ // 2, diffZ - diffZ // 2))
x = torch.cat([x2, x1], dim=1)
return self.conv(x)
class OutConv3D(nn.Module):
def __init__(self, in_channels, out_channels):
super(OutConv3D, self).__init__()
self.conv = nn.Conv3d(in_channels, out_channels, kernel_size=1)
def forward(self, x):
return self.conv(x)
class LMNet3D(nn.Module):
def __init__(self, n_channels, n_classes, bilinear=True):
super(LMNet3D, self).__init__()
self.n_channels = n_channels
self.n_classes = n_classes
self.bilinear = bilinear
self.inc = DoubleConv3D(n_channels, 64)
self.down1 = Down3D(64, 128)
self.down2 = Down3D(128, 256)
self.down3 = Down3D(256, 512)
factor = 2 if bilinear else 1
self.down4 = Down3D(512, 1024 // factor)
self.up1 = Up3D(1024, 512 // factor, bilinear)
self.up2 = Up3D(512, 256 // factor, bilinear)
self.up3 = Up3D(256, 128 // factor, bilinear)
self.up4 = Up3D(128, 64, bilinear)
self.outc = OutConv3D(64, n_classes)
def forward(self, x):
x1 = self.inc(x)
x2 = self.down1(x1)
x3 = self.down2(x2)
x4 = self.down3(x3)
x5 = self.down4(x4)
x = self.up1(x5, x4)
x = self.up2(x, x3)
x = self.up3(x, x2)
x = self.up4(x, x1)
logits = self.outc(x)
return logits
```
该代码实现了3D-LMNet网络的结构,包括DoubleConv3D、Down3D、Up3D和OutConv3D四个模块以及LMNet3D整个网络。其中,DoubleConv3D用于进行两次卷积操作,Down3D用于进行下采样操作,Up3D用于进行上采样操作,OutConv3D用于最终输出分割结果。在LMNet3D中,inc代表输入层,down1-4代表四个下采样层,up1-4代表四个上采样层,outc代表输出层。在forward方法中,首先进行一次输入层的卷积操作,然后依次进行四个下采样和四个上采样操作,最后进行一次输出层的卷积操作,得到分割结果。
阅读全文
相关推荐
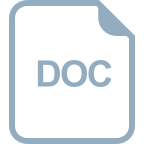














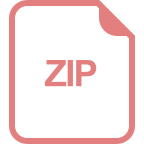
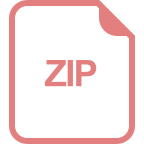
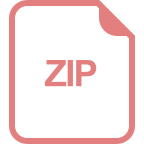