多线程实现mandelbrot
时间: 2024-02-03 20:02:01 浏览: 107
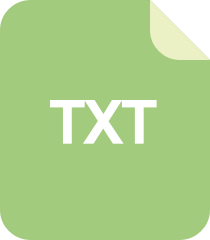
多线程实现
Mandelbrot集合是一个非常有趣的数学图形,可以使用多线程来实现它的绘制。下面是一个使用Python的多线程实现Mandelbrot集合的示例代码:
```python
import threading
import numpy as np
import matplotlib.pyplot as plt
def mandelbrot(xmin, xmax, ymin, ymax, width, height, max_iter):
x = np.linspace(xmin, xmax, width).reshape(1, width)
y = np.linspace(ymin, ymax, height).reshape(height, 1)
c = x + y * 1j
z = np.zeros_like(c)
for i in range(max_iter):
z = z**2 + c
mandelbrot_set = (abs(z) < 2)
return mandelbrot_set
def mandelbrot_thread(xmin, xmax, ymin, ymax, width, height, max_iter, row, result):
for i in range(row[0], row[1]):
result[i] = mandelbrot(xmin, xmax, ymin, ymax, width, 1, max_iter)[0]
def mandelbrot_multithread(xmin, xmax, ymin, ymax, width, height, max_iter, num_threads=4):
rows = np.array_split(range(height), num_threads)
result = [None] * height
threads = []
for i in range(num_threads):
t = threading.Thread(target=mandelbrot_thread, args=(xmin, xmax, ymin, ymax, width, height, max_iter, rows[i], result))
threads.append(t)
t.start()
for t in threads:
t.join()
mandelbrot_set = np.vstack(result)
return mandelbrot_set
if __name__ == '__main__':
xmin, xmax, ymin, ymax = -2, 1, -1.5, 1.5
width, height = 800, 600
max_iter = 100
mandelbrot_set = mandelbrot_multithread(xmin, xmax, ymin, ymax, width, height, max_iter)
plt.imshow(mandelbrot_set, cmap='hot', extent=[xmin, xmax, ymin, ymax])
plt.show()
```
这个示例代码使用了numpy和matplotlib库。首先,我们定义了一个单线程的函数mandelbrot,它接受Mandelbrot集合的参数,计算出每个点是否属于Mandelbrot集合,并返回一个布尔类型的数组。
然后,我们定义了一个多线程的函数mandelbrot_multithread。它将要绘制的图形划分成多行,每行分配给一个线程。每个线程计算它分配到的行的Mandelbrot集合,并将结果储存在一个列表中。
最后,我们使用numpy的vstack函数将每个线程计算的结果合并成一个二维数组,并使用matplotlib将其绘制出来。
注意,由于多线程的计算可能会导致不同线程之间的计算顺序不确定,因此绘制出来的图形可能会有一些小的变化。但这不会影响图形的正确性。
阅读全文
相关推荐
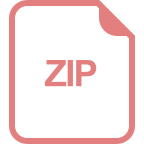
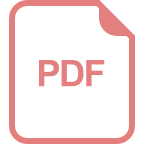
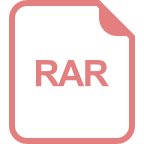
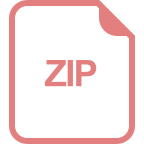
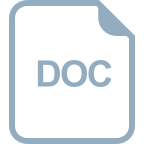
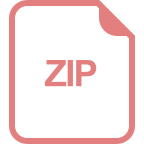
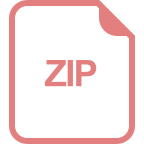
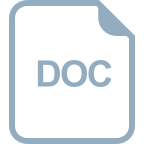
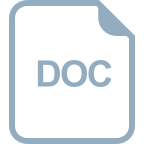
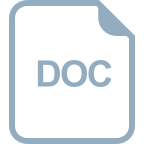
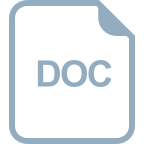
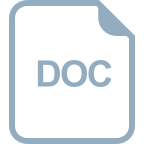
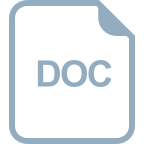
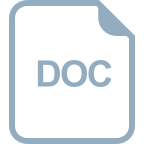
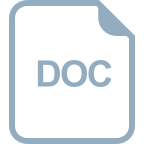
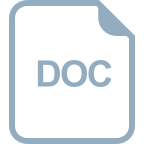
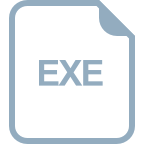