package Unit4; public class TwoThread implements Runnable{ // Class 'TwoThread' must either be declared abstract or implement abstract method 'run()' in 'Runnable' public int t; @Override public void run() { for (;t<10;t++){ //当线程类实现 Runnable 接口时 //如果想获取当前线程,只能用 Thread.currentThread() 方法 System.out.println(Thread.currentThread().getName()+" "+t); } } public static void main(String[] args) { for (int i = 0;i<10;i++){ System.out.println(Thread.currentThread().getName()+" "+i); if (i==5){ TwoThread tt = new TwoThread(); //创建并启动第一个线程 new Thread(tt,"新线程1").start(); //创建并启动第二个线程 new Thread(tt,"新线程2").start(); } } } }
时间: 2024-02-14 11:14:15 浏览: 20
这段代码中,创建了一个实现了Runnable接口的线程类TwoThread,实现了run()方法。在run()方法中,使用循环输出线程名称和循环变量t的值。在main()方法中,使用循环输出主线程的名称和循环变量i的值,当i等于5时,创建了一个TwoThread对象tt,并启动了两个新线程,都使用了同一个tt对象,因此会输出两个新线程的信息,但是它们都在执行同一个run()方法,因此输出的内容是相同的。在输出线程信息时,使用了Thread.currentThread().getName()方法获取当前线程的名称。
相关问题
编写线程类DelayPrintThread,构造方法中给出线程号,在线程体中产生一个1-10之间的随机数,使得线程体每休眠此随机数时间就打印输出线程号和休眠时间;另外编写应用DelayPrintThread类的Java应用程序TwoThread.java,在main()方法中创建两个线程,并应用sleep()控制主应用程序延迟一段时间。
好的,我来帮您编写。
DelayPrintThread类的代码如下:
```java
public class DelayPrintThread extends Thread {
private int threadNumber;
public DelayPrintThread(int threadNumber) {
this.threadNumber = threadNumber;
}
public void run() {
try {
while (true) {
int sleepTime = (int) (Math.random() * 10) + 1;
Thread.sleep(sleepTime * 1000);
System.out.println("Thread " + threadNumber + " slept for " + sleepTime + " seconds.");
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
TwoThread类的代码如下:
```java
public class TwoThread {
public static void main(String[] args) {
DelayPrintThread thread1 = new DelayPrintThread(1);
DelayPrintThread thread2 = new DelayPrintThread(2);
thread1.start();
thread2.start();
try {
Thread.sleep(10000); // 主线程延迟10秒钟
} catch (InterruptedException e) {
e.printStackTrace();
}
thread1.interrupt();
thread2.interrupt();
}
}
```
在TwoThread类中,我们创建了两个DelayPrintThread对象,并启动它们的线程。然后,主线程使用sleep()方法延迟10秒钟,之后调用线程的interrupt()方法来终止线程的执行。
执行TwoThread类,可以看到两个线程交替输出线程号和休眠时间。当主线程执行完毕后,两个子线程也会被终止。
相关推荐
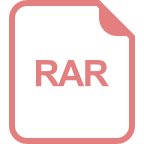
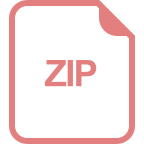
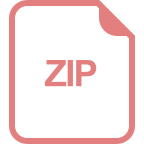
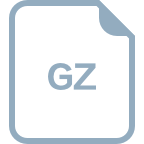
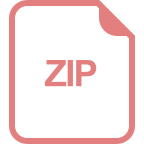
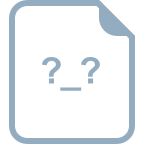
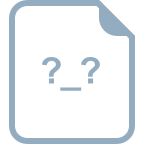
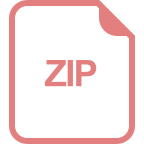
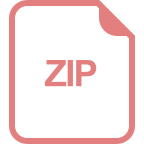
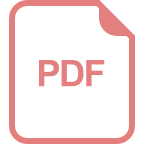
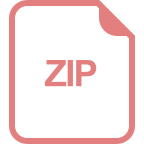
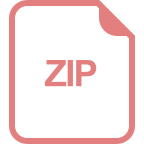