利用C++编写并测试程序:(输入输出流程序操作) 用户从键盘输入若干行字符串,每输入一行就将其写入一个二进制文件中,直到用户输入“end”才终止输入。输入完毕后,再从二进制文件中按输入的行号读取数据并输出到屏幕上。
时间: 2024-03-18 20:42:39 浏览: 70
好的,这是一个比较复杂的程序,我会尽量给您详细解释代码实现过程。以下是 C++ 代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
fstream file("data.bin", ios::out | ios::binary);
// 以写入二进制模式打开文件
if (!file) {
cerr << "Failed to open file!" << endl;
return 1;
}
string input;
int line = 1;
while (true) {
cout << "Please enter a line of text (or 'end' to finish):" << endl;
getline(cin, input);
if (input == "end") {
break;
}
file.write(reinterpret_cast<const char*>(&line), sizeof(line));
// 将行号写入文件
int length = input.length();
file.write(reinterpret_cast<const char*>(&length), sizeof(length));
// 将字符串长度写入文件
file.write(input.c_str(), length);
// 将字符串写入文件
line++;
}
file.close();
// 关闭文件
file.open("data.bin", ios::in | ios::binary);
// 以读取二进制模式打开文件
if (!file) {
cerr << "Failed to open file!" << endl;
return 1;
}
file.seekg(0, ios::end);
int length = file.tellg();
file.seekg(0, ios::beg);
// 获取文件长度并将文件指针移动到文件开头
while (file.tellg() < length) {
int currentLine;
file.read(reinterpret_cast<char*>(¤tLine), sizeof(currentLine));
// 读取行号
int currentLength;
file.read(reinterpret_cast<char*>(¤tLength), sizeof(currentLength));
// 读取字符串长度
char* buffer = new char[currentLength + 1];
file.read(buffer, currentLength);
// 读取字符串
buffer[currentLength] = '\0';
cout << "Line " << currentLine << ": " << buffer << endl;
delete[] buffer;
}
file.close();
// 关闭文件
return 0;
}
```
这个程序首先打开一个文件 `data.bin`,并以写入二进制模式打开。然后,它进入一个循环,提示用户输入一行文本。每次用户输入一行文本,程序就会将该行文本写入文件,并在文件中添加一个行号。行号从 1 开始,每次用户输入一行文本,行号就会递增。
用户可以输入“end”结束输入过程,此时程序会关闭文件。
然后,程序再次打开文件,这次以读取二进制模式打开。它使用 `seekg` 函数将文件指针移动到文件开头,然后读取每一行文本。每一行文本的格式为:4 个字节的行号 + 4 个字节的字符串长度 + 字符串。
程序通过读取行号和字符串长度来确定每一行文本在文件中的位置。然后,它使用 `new` 运算符动态分配一个 char 数组,并使用 `read` 函数从文件中读取字符串。最后,程序将字符串输出到屏幕上,并使用 `delete[]` 运算符释放 char 数组。
最后,程序关闭文件并退出。
阅读全文
相关推荐
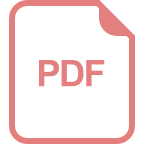
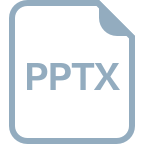
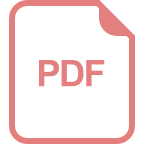
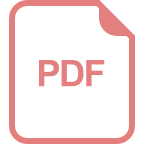
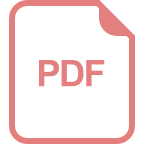
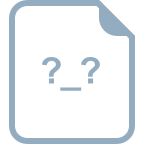
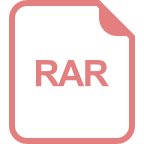
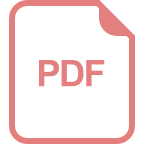
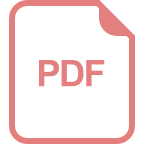
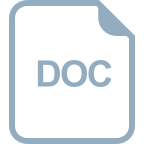
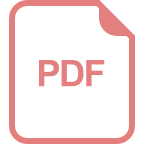
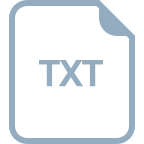
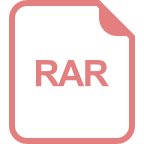
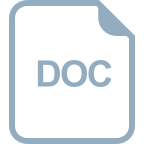
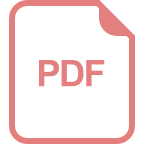