python 第k大
时间: 2024-06-16 08:06:22 浏览: 98
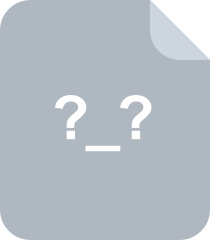
python 实现第k个排列
在Python中,可以使用不同的方法来找到一个列表中的第k大元素。以下是一种常见的方法:
1. 使用内置函数sorted():可以使用sorted()函数对列表进行排序,并返回一个新的已排序列表。然后,可以通过索引来获取第k大的元素。
示例代码:
```python
def find_kth_largest(nums, k):
sorted_nums = sorted(nums, reverse=True)
return sorted_nums[k-1]
```
2. 使用堆数据结构:可以使用heapq模块中的nlargest()函数来找到列表中的第k大元素。该函数会返回一个列表,其中包含了前k个最大的元素。
示例代码:
```python
import heapq
def find_kth_largest(nums, k):
return heapq.nlargest(k, nums)[-1]
```
3. 使用快速选择算法:快速选择算法是一种基于快速排序思想的算法,可以在平均情况下以线性时间复杂度找到第k大元素。
示例代码:
```python
import random
def partition(nums, low, high):
pivot_index = random.randint(low, high)
pivot = nums[pivot_index]
nums[pivot_index], nums[high] = nums[high], nums[pivot_index]
i = low
for j in range(low, high):
if nums[j] >= pivot:
nums[i], nums[j] = nums[j], nums[i]
i += 1
nums[i], nums[high] = nums[high], nums[i]
return i
def find_kth_largest(nums, k):
low, high = 0, len(nums) - 1
while True:
pivot_index = partition(nums, low, high)
if pivot_index == k - 1:
return nums[pivot_index]
elif pivot_index > k - 1:
high = pivot_index - 1
else:
low = pivot_index + 1
```
阅读全文
相关推荐
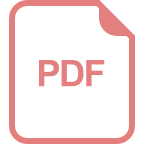
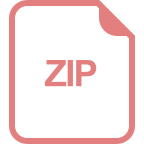
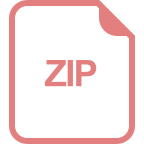
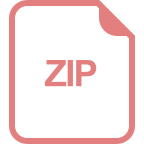
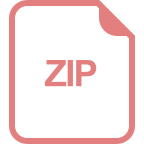
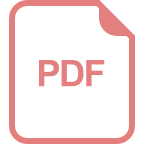
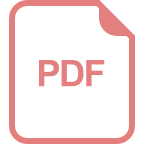
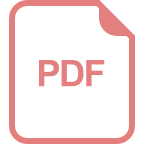
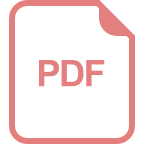
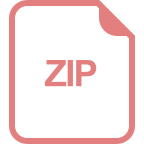
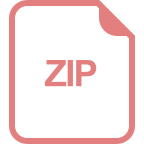
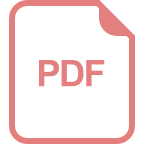
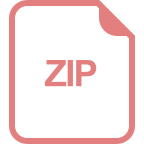