对Revit中所建立的三维模型信息进行提取、导出为JSON格式文件。
时间: 2024-05-01 10:21:05 浏览: 335
Revit是一种建筑信息模型(BIM)软件,它可以创建三维建筑模型。要将Revit中的三维模型信息提取并导出为JSON格式文件,您可以使用Revit API和JSON.NET库。
以下是大体步骤:
1. 创建一个C#类库项目,并添加对RevitAPI和JSON.NET库的引用。
2. 在Visual Studio中打开Revit,创建一个新的命令类。
3. 在命令类中,使用FilteredElementCollector类来选择您想要提取信息的元素。例如,您可以选择所有墙体元素。
4. 遍历所选元素,并将所需的属性值保存到一个自定义的类中。
5. 使用JSON.NET库将类序列化为JSON格式,并将其保存到文件中。
下面是一个简单的示例代码,演示如何将Revit中的墙体元素信息导出为JSON格式文件:
```csharp
using Autodesk.Revit.Attributes;
using Autodesk.Revit.DB;
using Autodesk.Revit.UI;
using Newtonsoft.Json;
using System.Collections.Generic;
using System.IO;
[Transaction(TransactionMode.Manual)]
public class ExportToJsonCommand : IExternalCommand
{
public Result Execute(ExternalCommandData commandData, ref string message, ElementSet elements)
{
// 获取当前文档
Document doc = commandData.Application.ActiveUIDocument.Document;
// 创建一个墙体信息列表
List<WallInfo> wallInfos = new List<WallInfo>();
// 选择所有墙体元素
FilteredElementCollector collector = new FilteredElementCollector(doc);
ICollection<Element> walls = collector.OfClass(typeof(Wall)).ToElements();
// 遍历所有墙体元素,并将其信息保存到WallInfo类中
foreach (Wall wall in walls)
{
WallInfo wallInfo = new WallInfo();
wallInfo.Id = wall.Id.IntegerValue;
wallInfo.Name = wall.Name;
wallInfo.Width = wall.Width;
wallInfo.Height = wall.Height;
wallInfos.Add(wallInfo);
}
// 将墙体信息列表序列化为JSON格式
string json = JsonConvert.SerializeObject(wallInfos, Formatting.Indented);
// 将JSON格式数据保存到文件中
string filePath = "C:\\Temp\\walls.json";
File.WriteAllText(filePath, json);
return Result.Succeeded;
}
}
// 自定义的墙体信息类
public class WallInfo
{
public int Id { get; set; }
public string Name { get; set; }
public double Width { get; set; }
public double Height { get; set; }
}
```
在此示例代码中,我们选择所有墙体元素,并将其信息保存到WallInfo类中。然后,使用JSON.NET库将WallInfo列表序列化为JSON格式,并将其保存到文件中。在此示例中,我们将文件保存到“C:\Temp\walls.json”路径下。
您可以根据自己的需要修改此代码,以提取和导出所需的Revit元素信息。
阅读全文
相关推荐
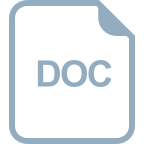
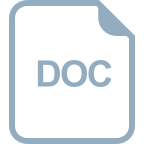
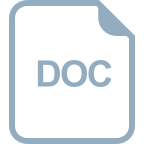
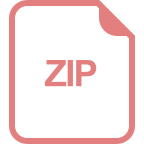
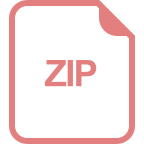
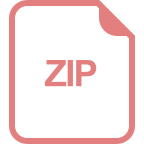
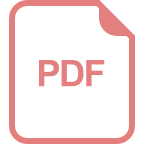
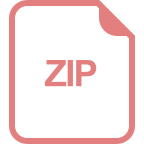
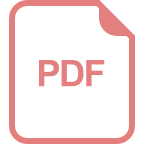
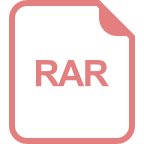
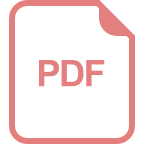
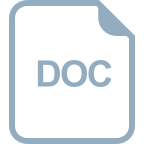
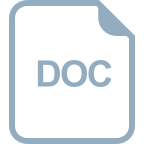

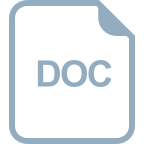
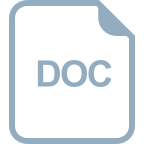
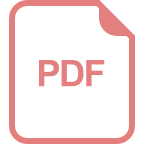
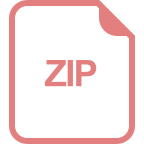
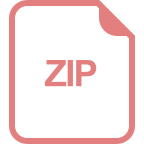