matcher中appendReplacement方法如何用c++实现
时间: 2023-11-22 22:54:57 浏览: 21
在 C++ 中,可以使用正则表达式库 `regex` 来实现 `appendReplacement` 方法的功能。具体实现步骤如下:
1. 定义一个正则表达式对象和一个字符串,用于存储匹配结果和替换后的字符串:
```
std::regex pattern("匹配的正则表达式");
std::string input("输入的字符串");
std::string output;
```
2. 使用 `std::sregex_iterator` 遍历字符串中所有匹配的子串,并在循环中使用 `std::regex_replace` 方法替换匹配结果:
```
std::sregex_iterator it(input.begin(), input.end(), pattern);
std::sregex_iterator end;
int last_pos = 0;
for (; it != end; ++it) {
std::smatch match = *it;
int pos = match.position();
output.append(input, last_pos, pos - last_pos);
// 进行替换操作
// ...
last_pos = pos + match.length();
}
output.append(input, last_pos, input.length() - last_pos);
```
3. 在循环中,可以使用 `std::regex_replace` 方法将匹配结果替换为指定的字符串:
```
std::string replacement("替换的字符串");
std::string result = std::regex_replace(match.str(), pattern, replacement);
output.append(result);
```
完整代码示例:
```
#include <regex>
#include <string>
#include <iostream>
int main() {
std::regex pattern("(\\w+)");
std::string input("hello world");
std::string output;
std::sregex_iterator it(input.begin(), input.end(), pattern);
std::sregex_iterator end;
int last_pos = 0;
for (; it != end; ++it) {
std::smatch match = *it;
int pos = match.position();
output.append(input, last_pos, pos - last_pos);
std::string replacement("replacement");
std::string result = std::regex_replace(match.str(), pattern, replacement);
output.append(result);
last_pos = pos + match.length();
}
output.append(input, last_pos, input.length() - last_pos);
std::cout << output << std::endl;
return 0;
}
```
相关推荐
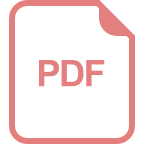
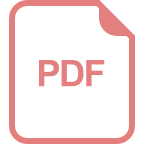










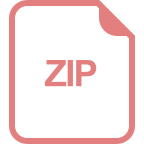