C语言MySQL增删查改
时间: 2023-12-05 13:04:44 浏览: 45
在C语言中,我们可以使用MySQL提供的API来实现MySQL数据库的增删查改操作。以下是一些基本的MySQL操作示例:
1. 连接MySQL数据库
```c
#include <mysql.h>
MYSQL *conn;
conn = mysql_init(NULL);
/* 连接数据库 */
if (!mysql_real_connect(conn, "localhost", "root", "password", "database_name", 0, NULL, 0)) {
printf("%s\n", mysql_error(conn));
exit(1);
}
```
2. 插入数据
```c
char *query;
query = "INSERT INTO table_name (column1, column2, ...) VALUES ('value1', 'value2', ...)";
if (mysql_query(conn, query)) {
printf("%s\n", mysql_error(conn));
exit(1);
}
```
3. 查询数据
```c
MYSQL_RES *result;
MYSQL_ROW row;
char *query;
int num_fields;
query = "SELECT * FROM table_name";
if (mysql_query(conn, query)) {
printf("%s\n", mysql_error(conn));
exit(1);
}
result = mysql_store_result(conn);
num_fields = mysql_num_fields(result);
while ((row = mysql_fetch_row(result))) {
for(int i = 0; i < num_fields; i++) {
printf("%s ", row[i] ? row[i] : "NULL");
}
printf("\n");
}
mysql_free_result(result);
```
4. 更新数据
```c
char *query;
query = "UPDATE table_name SET column1='value1', column2='value2', ... WHERE condition";
if (mysql_query(conn, query)) {
printf("%s\n", mysql_error(conn));
exit(1);
}
```
5. 删除数据
```c
char *query;
query = "DELETE FROM table_name WHERE condition";
if (mysql_query(conn, query)) {
printf("%s\n", mysql_error(conn));
exit(1);
}
```
注意:以上代码示例仅供参考,实际使用时需要根据具体情况进行修改。同时,在使用MySQL API操作数据库时,需要注意SQL注入攻击等安全问题。
阅读全文
相关推荐
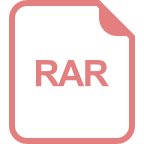
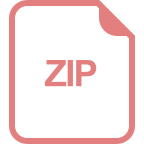
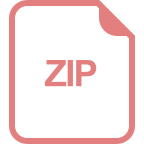
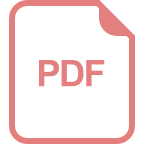
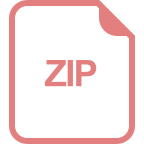
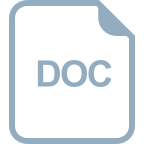
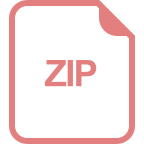
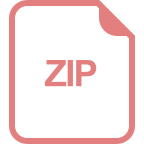
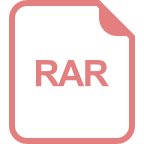
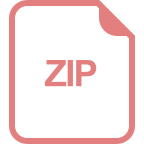
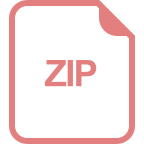
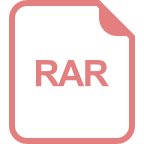
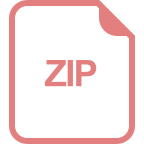
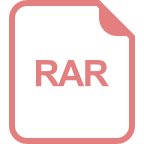
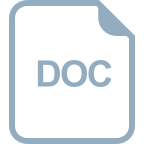
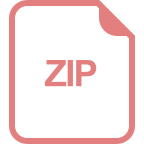
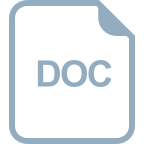