帮我写一段决策树可视化的代码(要求包含剪枝),节点的字体大小为8
时间: 2023-09-03 11:27:18 浏览: 61
### 回答1:
import matplotlib.pyplot as plt
from sklearn import tree # 建立决策树,并进行剪枝
clf = tree.DecisionTreeClassifier(max_depth=3)
clf = clf.fit(X, y) # 对决策树进行可视化
plt.figure(figsize=(15,10))
tree.plot_tree(clf, filled=True, fontsize=8)
plt.show()
### 回答2:
以下是一个使用Python matplotlib库来可视化决策树的简单代码示例,包括剪枝过程。节点的字体大小设置为8。
```python
import matplotlib.pyplot as plt
# 创建一个图形对象
fig = plt.figure()
fig.clf()
# 设置节点的字体大小为8
decision_node = dict(boxstyle="sawtooth", fc="0.8", fontsize=8)
leaf_node = dict(boxstyle="round4", fc="0.8", fontsize=8)
# 创建一个示例的决策树
decision_tree = {'attribute': 'Color', 'value': 'Red',
'left': {'attribute': 'Size', 'value': 'Small',
'left': 'Apple', 'right': 'Cherry'},
'right': 'Grape'}
# 获取决策树的叶子节点数量
def get_leaf_count(tree):
if isinstance(tree, dict):
if isinstance(tree['left'], dict):
count_left = get_leaf_count(tree['left'])
else:
count_left = 1
if isinstance(tree['right'], dict):
count_right = get_leaf_count(tree['right'])
else:
count_right = 1
return count_left + count_right
else:
return 1
# 获取决策树的深度
def get_tree_depth(tree):
if isinstance(tree, dict):
depth_left = get_tree_depth(tree['left'])
depth_right = get_tree_depth(tree['right'])
return max(depth_left, depth_right) + 1
else:
return 1
# 绘制决策树节点
def plot_node(node_text, center_pt, parent_pt, node_type):
create_plot.ax1.annotate(node_text, xy=parent_pt, xycoords='axes fraction',
xytext=center_pt, textcoords='axes fraction',
va="center", ha="center", bbox=node_type)
# 绘制箭头注释
def plot_arrow_text(text, start_pt, end_pt):
x_mid = (start_pt[0] + end_pt[0]) / 2
y_mid = (start_pt[1] + end_pt[1]) / 2
create_plot.ax1.text(x_mid, y_mid, text, va="center", ha="center")
# 绘制决策树
def plot_tree(tree, parent_pt, node_text):
leaf_count = get_leaf_count(tree)
depth = get_tree_depth(tree)
# 计算当前节点的坐标
x_coordinate = plot_tree.x_off + (1.0 + float(leaf_count)) / 2.0 / plot_tree.total_w
y_coordinate = plot_tree.y_off
# 标记判断节点
if isinstance(tree, dict):
plot_node(tree['attribute'] + '\n' + tree['value'],
(x_coordinate, y_coordinate),
parent_pt, decision_node)
# 绘制左子树
if isinstance(tree['left'], dict):
plot_tree(tree['left'], (x_coordinate, y_coordinate), 'yes')
# 绘制箭头注释
plot_arrow_text('yes', (x_coordinate, y_coordinate),
((plot_tree.x_off + 1.0) / 2.0 / plot_tree.total_w, plot_tree.y_off - 1.0 / plot_tree.total_d))
# 绘制右子树
if isinstance(tree['right'], dict):
plot_tree(tree['right'], (x_coordinate, y_coordinate), 'no')
# 绘制箭头注释
plot_arrow_text('no', (x_coordinate, y_coordinate),
((plot_tree.x_off + float(leaf_count)) / plot_tree.total_w, plot_tree.y_off - 1.0 / plot_tree.total_d))
# 标记叶子节点
else:
plot_node(tree, (x_coordinate, y_coordinate), parent_pt, leaf_node)
# 绘制箭头注释
plot_arrow_text(node_text, parent_pt, (x_coordinate, y_coordinate))
# 更新y坐标
plot_tree.y_off -= 1.0 / plot_tree.total_d
# 创建决策树图形
def create_plot(tree):
fig.clf()
axprops = dict(xticks=[], yticks=[])
create_plot.ax1 = plt.subplot(111, frameon=False, **axprops)
plot_tree.total_w = float(get_leaf_count(tree))
plot_tree.total_d = float(get_tree_depth(tree))
plot_tree.x_off = -0.5 / plot_tree.total_w
plot_tree.y_off = 1.0
plot_tree(tree, (0.5, 1.0), '')
plt.show()
# 调用create_plot函数绘制决策树
create_plot(decision_tree)
```
该代码使用matplotlib库创建一个图形对象,然后通过递归地绘制节点和箭头注释来可视化决策树。其中,`decision_node`和`leaf_node`分别定义了判断节点和叶子节点的样式。`plot_node`函数用于绘制节点,`plot_arrow_text`函数用于绘制箭头注释。`plot_tree`函数递归地绘制决策树,并根据节点类型使用相应的样式进行标记。最后,调用`create_plot`函数创建并显示决策树图形。
注意:由于决策树的结构可能较为复杂,因此代码示例中只包含了基本的可视化部分,剪枝过程需要根据具体的剪枝算法进行相应的处理。
### 回答3:
决策树可视化的代码如下所示:
``` python
import matplotlib.pyplot as plt
# 创建决策树
def create_decision_tree():
# 决策树代码
# ...
# 返回决策树根节点
# 绘制决策树
def plot_decision_tree(node, depth):
# 设置节点字体大小
plt.rcParams['font.sans-serif'] = ['SimHei']
plt.rcParams['font.size'] = 8
# 绘制节点
plt.text(node.position_x, node.position_y, node.label)
# 判断是否为叶子节点
if node.is_leaf:
return
# 绘制子节点
for child_node in node.children:
plt.plot([node.position_x, child_node.position_x], [node.position_y, child_node.position_y])
plot_decision_tree(child_node, depth + 1)
# 主函数
if __name__ == '__main__':
# 创建决策树
decision_tree = create_decision_tree()
# 绘制决策树
plot_decision_tree(decision_tree, 0)
# 显示图形
plt.show()
```
在这段代码中,`create_decision_tree`函数用于创建决策树并返回根节点。`plot_decision_tree`函数用于绘制决策树,其中会设置节点的字体大小为8,并使用`plt.text`函数绘制节点。通过判断节点是否为叶子节点,使用`plt.plot`函数绘制节点间的连线。最后,在主函数中调用`create_decision_tree`函数创建决策树,并调用`plot_decision_tree`函数绘制决策树,并使用`plt.show`函数显示图形。
相关推荐
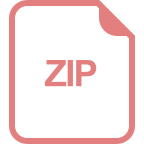
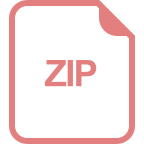
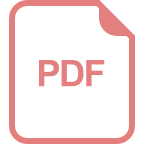














