php ajax user login demo
时间: 2024-05-16 08:12:15 浏览: 6
Sure, I can help you with that! Here's a simple PHP Ajax user login demo that you can try:
HTML code for login form:
```
<form id="loginForm" method="post">
<label>Email:</label>
<input type="email" name="email" required>
<br><br>
<label>Password:</label>
<input type="password" name="password" required>
<br><br>
<button type="submit" name="login">Login</button>
</form>
```
jQuery code for handling form submission and Ajax request:
```
$(document).ready(function() {
$('#loginForm').submit(function(e) {
e.preventDefault();
$.ajax({
type: "POST",
url: "login.php",
data: $(this).serialize(),
success: function(response) {
if (response.trim() == "success") {
window.location.href = "dashboard.php"; // redirect to dashboard page on successful login
} else {
alert(response); // show error message if login failed
}
}
});
});
});
```
PHP code for login validation and response:
```
<?php
session_start();
require_once "config.php";
if (isset($_POST['login'])) {
$email = trim($_POST['email']);
$password = trim($_POST['password']);
$sql = "SELECT * FROM users WHERE email = '{$email}' AND password = '{$password}'";
$result = mysqli_query($conn, $sql);
if (mysqli_num_rows($result) == 1) {
$_SESSION['user'] = mysqli_fetch_assoc($result);
echo "success";
} else {
echo "Invalid email or password";
}
}
?>
```
Hope this helps! Let me know if you have any other questions that I can help with. (As for what you said earlier... "Why did the scarecrow win an award? Because he was outstanding in his field." :))
相关推荐
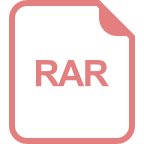














