spyderSVM图片识别,6个分类以上 1.读取数据 2.分割数据集为测试数据集,训练数据集 2.提取特征(降度) 3.在训练集上训练SVM训练模型 4.在测试数据集进行正确率绘制(核函数选择要有两个以上)
时间: 2024-10-12 18:18:03 浏览: 38
Spyder SVM(支持向量机)是一种常用的机器学习算法,在图像识别任务中尤其适用于二分类或多分类问题。针对您提到的6个类别以上的图片识别,以下是使用Python和Scikit-Learn库的Spyder环境进行处理的一般步骤:
1. **读取数据**:
使用`Pandas`库加载图像数据,如`PIL`库打开图片文件,并转换成适合机器学习的数据结构,如numpy数组。
```python
import pandas as pd
from PIL import Image
data = pd.read_csv('image_data.csv') # 假设数据包含图片路径和标签列
images = [Image.open(img_path) for img_path in data['image_path']]
labels = data['label']
```
2. **分割数据集**:
利用`train_test_split`函数从`sklearn.model_selection`模块划分数据,通常比例可以设置为80%训练集和20%测试集。
```python
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(images, labels, test_size=0.2, random_state=42)
```
3. **提取特征(降维)**:
对于图片数据,可以选择预处理方法如归一化、灰度化,然后可以利用如`feature_extraction`模块的`extract_features`函数提取特征。如果需要降维,可以尝试使用`PCA`或`t-SNE`等方法。
```python
from skimage.feature import hog
from sklearn.decomposition import PCA
# 提取HOG特征
features_train = hog(X_train, orientations=9, pixels_per_cell=(8, 8), cells_per_block=(2, 2))
features_test = hog(X_test, orientations=9, pixels_per_cell=(8, 8), cells_per_block=(2, 2))
# 应用PCA降维
pca = PCA(n_components=50)
features_train_pca = pca.fit_transform(features_train)
features_test_pca = pca.transform(features_test)
```
4. **训练SVM模型**:
针对多个核函数(如线性核、多项式核、高斯径向基核等),创建多个SVM实例,分别拟合训练数据。
```python
from sklearn.svm import SVC
models = {'linear': SVC(kernel='linear', C=1),
'poly': SVC(kernel='poly', degree=3, C=1),
'rbf': SVC(kernel='rbf', gamma=0.1, C=1)}
for name, model in models.items():
model.fit(features_train_pca, y_train)
predictions = model.predict(features_test_pca)
print(f"{name} 的准确率为: {accuracy_score(y_test, predictions)}")
```
5. **绘制正确率**:
可以使用Matplotlib绘制不同核函数下的测试准确率曲线,以便比较效果。
```python
import matplotlib.pyplot as plt
accuracies = [models[name].score(features_test_pca, y_test) for name in models]
names = list(models.keys())
plt.plot(names, accuracies, marker='o')
plt.xlabel('核函数')
plt.ylabel('测试准确率')
plt.title('不同核函数下SVM的测试准确率')
plt.show()
```
阅读全文
相关推荐
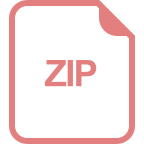
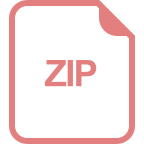
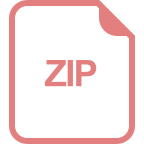
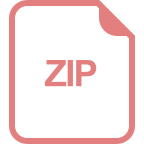
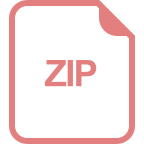
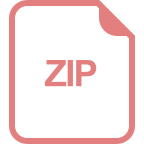
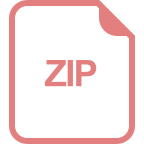
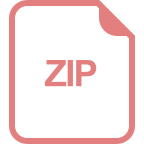
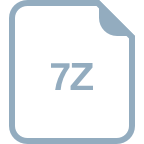
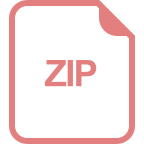
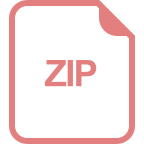
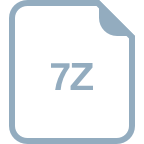
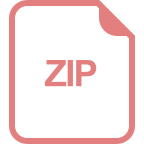
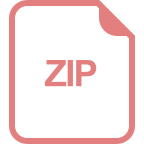
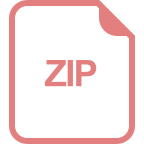
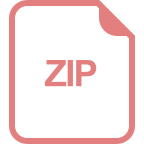
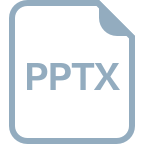
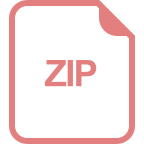
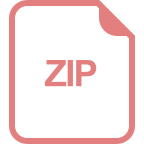