C#实现ssh远程linux时执行当前指令时,在当前进程运行时远程结束该进程
时间: 2024-11-09 15:24:00 浏览: 12
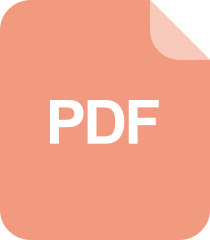
C#实现查杀本地与远程进程的方法
在C#中,要通过SSH(Secure Shell)连接到Linux服务器并终止运行中的进程,通常会使用第三方库如`SSH.NET`或`libssh2-net`。首先,确保已经安装了这些依赖。以下是一个简单的示例,展示如何使用`SSH.NET`来实现这一功能:
```csharp
using SSH.NET;
public void KillRemoteProcess(string serverIp, string username, string password, int processId)
{
using (var client = new SshClient(serverIp))
{
// 设置SSH连接信息
client.ConnectionInfo.AuthenticationMethod = AuthenticationMethod.Password;
client.ConnectionInfo.Username = username;
client.ConnectionInfo.Password = password;
try
{
// 连接到服务器
client.Connect();
// 获取Shell通道
using (var shell = client.CreateShell())
{
// 发送命令(假设`ps aux | grep <processName>`能找到进程ID)
var command = $"ps aux | grep {(processId)} | awk '{{print $2}}'";
var output = shell.Run(command);
// 如果找到了进程,发送kill命令
if (!string.IsNullOrEmpty(output.StandardError))
{
var pidLine = output.StandardError.ReadLine();
if (!string.IsNullOrWhiteSpace(pidLine) && int.TryParse(pidLine, out int remotePid))
{
var killCommand = $"kill -9 {remotePid}";
shell.Run(killCommand);
Console.WriteLine($"远程进程 {processId} 已经终止");
}
else
{
Console.WriteLine("未找到指定进程或无法获取PID");
}
}
else
{
Console.WriteLine("未找到指定进程");
}
// 关闭shell通道
shell.Disconnect();
}
}
catch (Exception ex)
{
Console.WriteLine($"错误:{ex.Message}");
client.Disconnect();
}
}
}
```
在这个例子中,你需要替换`serverIp`, `username`, `password`以及`processId`为你实际的值,并且如果需要根据其他条件查找进程,你可以修改`command`变量中的grep查询。
阅读全文
相关推荐
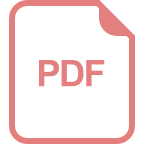
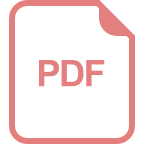
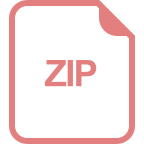
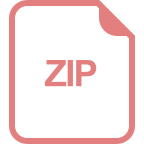
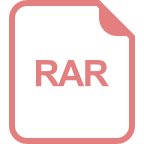
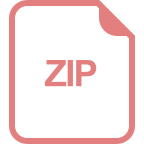
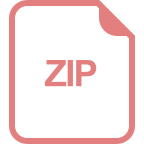
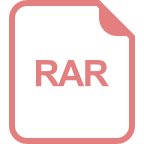
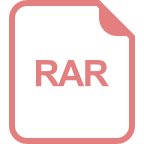
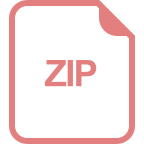
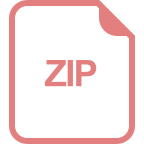
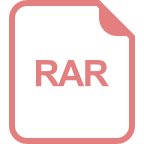
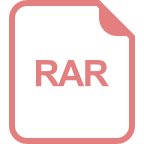
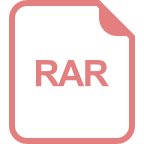
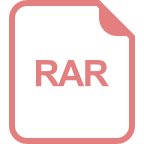
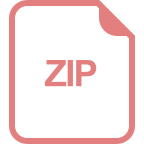
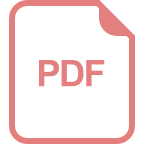