public String getLogin(String identityNum, String password) { String name=null; ResultSet rs=null; String sql="select patientName from patient where identityNum=? and password=?"; try { if (rs!=null) { rs = executeQuery(sql, identityNum, password); if (rs.next()) { name =rs.getString("patientName"); } } }catch (SQLException throwables) { throwables.printStackTrace(); } return name; }
时间: 2024-02-15 07:28:20 浏览: 57
这段代码看起来是一个Java方法,接收两个参数:身份证号码和密码,然后查询数据库中是否存在符合条件的病人信息。如果查询成功,返回病人姓名;否则返回null。
其中,executeQuery方法执行了一个SQL查询语句,返回一个结果集ResultSet。如果结果集不为空,通过rs.getString("patientName")获取病人姓名。
需要注意的是,这段代码中存在一个问题:没有对rs进行null值判断,可能会导致空指针异常。应该在执行rs之前加上一个判断,如:
if(rs != null) {
// execute rs
} else {
// handle exception
}
相关问题
<div id="page_login"> <div class="loginContainer"> <div style="font-size: 36px;margin-bottom: 20px;text-align: center;font-family: 'Lucida Sans', 'Lucida Sans Regular', 'Lucida Grande', 'Lucida Sans Unicode', Geneva, Verdana, sans-serif;"> Login</div> <input type="text" name="account" id="account" placeholder="account"> <input type="password" name="password" id="password" placeholder="password"> <button id="loginButton" onclick="getLogin()">login</button> </div> </div>
这段代码是一个包含登录表单的HTML页面,其中包括一个账号输入框、一个密码输入框和一个登录按钮。当用户点击登录按钮时,会调用名为 `getLogin()` 的JavaScript函数进行登录验证。
你可以使用以下JavaScript代码来获取用户输入的账号和密码,并通过ajax向服务器发送登录请求:
```javascript
// 获取账号和密码输入框的引用
var accountInput = document.getElementById('account');
var passwordInput = document.getElementById('password');
// 获取登录按钮的引用
var loginButton = document.getElementById('loginButton');
// 绑定登录事件处理函数
loginButton.addEventListener('click', function() {
// 获取用户输入的账号和密码
var account = accountInput.value;
var password = passwordInput.value;
// 创建一个XMLHttpRequest对象
var xhr = new XMLHttpRequest();
// 设置请求参数
xhr.open('POST', 'your_login_url');
xhr.setRequestHeader('Content-Type', 'application/json');
// 将数据转换为JSON字符串
var data = {
account: account,
password: password
};
var json = JSON.stringify(data);
// 发送请求
xhr.send(json);
// 处理响应数据
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE) {
if (xhr.status === 200) {
var response = JSON.parse(xhr.responseText);
if (response.status === 'success') {
// 登录成功,跳转到主页面
window.location.href = 'your_main_page_url';
} else {
// 登录失败,显示错误信息
alert(response.message);
}
} else {
console.error('请求失败:', xhr.status);
}
}
};
});
```
在代码中,你需要将 `your_login_url` 替换为服务器端登录验证的接口地址,将 `your_main_page_url` 替换为登录成功后跳转到的主页面地址。在服务器端,你需要解析JSON字符串,并验证账号和密码是否正确,然后返回响应数据。
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <title>登录界面</title> <style> body { margin: 0; padding: 0; background-color: #f2f2f2; font-family: 'Lucida Sans', 'Lucida Sans Regular', 'Lucida Grande', 'Lucida Sans Unicode', Geneva, Verdana, sans-serif; } #page_login { display: flex; justify-content: center; align-items: center; height: 100vh; } .loginContainer { display: flex; flex-direction: column; align-items: center; justify-content: center; background-color: white; border-radius: 5px; box-shadow: 0px 0px 10px rgba(0,0,0,0.2); padding: 30px; } .loginContainer > div { font-size: 36px; margin-bottom: 20px; text-align: center; } input[type="text"], input[type="password"] { width: 100%; padding: 10px; margin-bottom: 10px; border: none; border-radius: 5px; box-shadow: 0px 0px 5px rgba(0,0,0,0.1); } #loginButton { background-color: #007bff; color: white; padding: 10px 20px; border: none; border-radius: 5px; box-shadow: 0px 0px 5px rgba(0,0,0,0.1); transition: all 0.2s ease-in-out; } #loginButton:hover { transform: scale(1.05); box-shadow: 0px 0px 10px rgba(0,0,0,0.2); } </style> </head> <body> <!-- 登录界面 --> <div id="page_login"> <div class="loginContainer"> <div>Login</div> <input type="text" name="account" id="account" placeholder="account"> <input type="password" name="password" id="password" placeholder="password"> <button id="loginButton" onclick="getLogin()">login</button> </div> <button id="checkinto">select</button> </div> </body> <script> document.getElementById('checkinto').addEventListener('click', function() { window.location.href = 'select.html'; }); </script> <script type="text/javascript" src="./js/index.js"></script> <script src="./js/jquery-3.3.1.js"></script> </html>美化这段代码
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>登录界面</title>
<style>
body {
margin: 0;
padding: 0;
background-color: #f2f2f2;
font-family: 'Lucida Sans', 'Lucida Sans Regular', 'Lucida Grande', 'Lucida Sans Unicode', Geneva, Verdana, sans-serif;
}
#page_login {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.loginContainer {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
background-color: white;
border-radius: 5px;
box-shadow: 0px 0px 10px rgba(0,0,0,0.2);
padding: 30px;
}
.loginContainer > div {
font-size: 36px;
margin-bottom: 20px;
text-align: center;
}
input[type="text"], input[type="password"] {
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: none;
border-radius: 5px;
box-shadow: 0px 0px 5px rgba(0,0,0,0.1);
}
#loginButton {
background-color: #007bff;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
box-shadow: 0px 0px 5px rgba(0,0,0,0.1);
transition: all 0.2s ease-in-out;
}
#loginButton:hover {
transform: scale(1.05);
box-shadow: 0px 0px 10px rgba(0,0,0,0.2);
}
</style>
</head>
<body>
<!-- 登录界面 -->
<div id="page_login">
<div class="loginContainer">
<div>Login</div>
<input type="text" name="account" id="account" placeholder="账号">
<input type="password" name="password" id="password" placeholder="密码">
<button id="loginButton" onclick="getLogin()">登录</button>
</div>
<button id="checkinto">选择</button>
</div>
</body>
<script>
document.getElementById('checkinto').addEventListener('click', function() {
window.location.href = 'select.html';
});
</script>
<script type="text/javascript" src="./js/index.js"></script>
<script src="./js/jquery-3.3.1.js"></script>
</html>
阅读全文
相关推荐
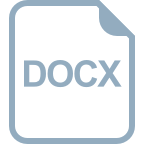
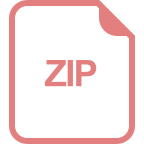








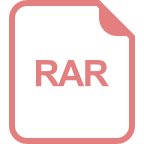
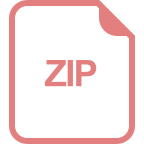
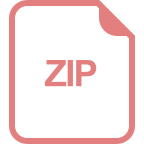
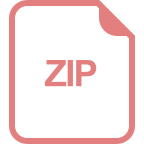