使用python分别采用网格搜索和随机搜索方法对KNN模型中的k值进行优化,具体解决鸢尾花分类问题,k取1到20之内的所有奇数。最后输出最优的模型、k值和多分类的各项性能指标、PR曲线和ROC曲线。
时间: 2024-04-22 09:27:31 浏览: 14
好的,那么我们来逐步实现这个需求。
首先,我们需要导入需要的库:sklearn用于机器学习模型,numpy用于数据处理,matplotlib用于可视化。请确认您的电脑中已经安装了它们,如果没有,请使用以下命令进行安装:
```
pip install scikit-learn numpy matplotlib
```
接下来,我们需要加载鸢尾花数据集。可以使用sklearn中自带的iris数据集,代码如下:
```python
from sklearn.datasets import load_iris
iris = load_iris()
X = iris.data
y = iris.target
```
接着,我们需要将数据集分成训练集和测试集。我们可以使用sklearn中的train_test_split函数:
```python
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
现在我们可以开始使用KNN模型进行分类。我们将使用sklearn中的KNeighborsClassifier类,代码如下:
```python
from sklearn.neighbors import KNeighborsClassifier
knn = KNeighborsClassifier()
```
根据需求,我们需要尝试不同的k值。我们可以使用网格搜索和随机搜索两种方法来找到最优的k值。
首先,我们来看网格搜索。我们需要指定需要尝试的参数和取值范围,代码如下:
```python
from sklearn.model_selection import GridSearchCV
param_grid = {'n_neighbors': list(range(1, 20, 2))}
grid_search = GridSearchCV(knn, param_grid, cv=5, scoring='accuracy')
grid_search.fit(X_train, y_train)
```
其中,param_grid是需要尝试的参数和取值范围,这里是k的取值范围。cv是交叉验证的折数,这里指定为5。scoring是评估指标,这里我们使用准确率(accuracy)。
接着,我们可以输出最优的模型、k值和各项性能指标,代码如下:
```python
print('Best model:', grid_search.best_estimator_)
print('Best k:', grid_search.best_params_['n_neighbors'])
print('Train accuracy:', grid_search.best_score_)
print('Test accuracy:', grid_search.score(X_test, y_test))
```
现在我们来看随机搜索。和网格搜索类似,我们需要指定需要尝试的参数和取值范围,代码如下:
```python
from sklearn.model_selection import RandomizedSearchCV
param_distributions = {'n_neighbors': list(range(1, 20, 2))}
random_search = RandomizedSearchCV(knn, param_distributions, cv=5, scoring='accuracy', n_iter=10, random_state=42)
random_search.fit(X_train, y_train)
```
其中,param_distributions是需要尝试的参数和取值范围,这里是k的取值范围。n_iter是随机搜索的次数,这里指定为10。其他参数的含义和网格搜索相同。
接着,我们可以输出最优的模型、k值和各项性能指标,代码如下:
```python
print('Best model:', random_search.best_estimator_)
print('Best k:', random_search.best_params_['n_neighbors'])
print('Train accuracy:', random_search.best_score_)
print('Test accuracy:', random_search.score(X_test, y_test))
```
最后,我们可以输出PR曲线和ROC曲线。代码如下:
```python
from sklearn.metrics import plot_precision_recall_curve, plot_roc_curve
import matplotlib.pyplot as plt
fig, ax = plt.subplots(1, 2, figsize=(12, 6))
plot_precision_recall_curve(grid_search.best_estimator_, X_test, y_test, ax=ax[0])
ax[0].set_title('PR Curve')
plot_roc_curve(grid_search.best_estimator_, X_test, y_test, ax=ax[1])
ax[1].set_title('ROC Curve')
plt.show()
```
这里我们使用了sklearn中的plot_precision_recall_curve和plot_roc_curve函数来绘制PR曲线和ROC曲线。需要注意的是,这里的模型需要使用网格搜索或随机搜索得到的最优模型。
综上,我们完成了对KNN模型中的k值进行优化,并输出了最优的模型、k值和各项性能指标、PR曲线和ROC曲线。
相关推荐
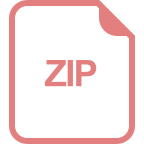
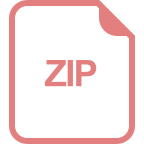






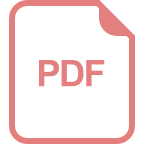
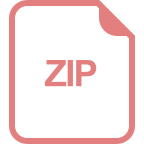
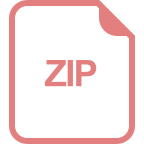
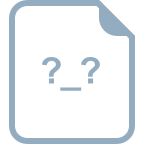
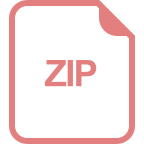