features, labels = get_rolling_window_multistep(output_length, 0, input_length, features_.T, np.expand_dims(labels_, 0))
时间: 2023-06-12 07:04:13 浏览: 143
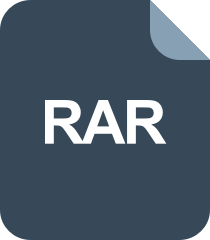
knn.rar_K._KNN K_knn_knn matlab
这行代码的作用是获取滚动窗口多步预测的特征和标签。其中,`output_length`表示预测的时间步长,`input_length`表示输入的时间步长,`features_.T`表示特征数据的转置,`np.expand_dims(labels_, 0)`表示标签数据的维度扩展。该函数将滚动窗口的特征和标签以元组的形式返回。
阅读全文
相关推荐
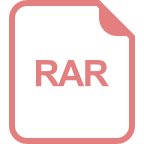
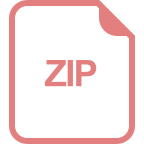



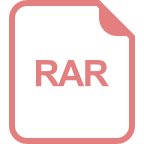
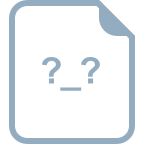
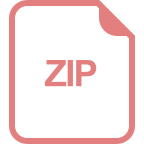
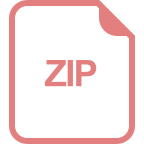
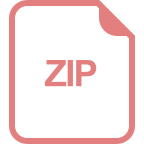
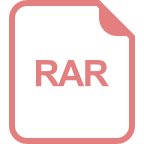
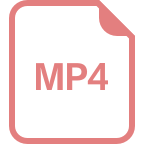
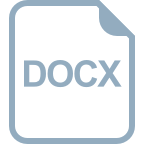
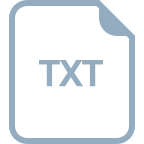