matlab中找出两个cell中字符串一样
时间: 2024-09-11 08:18:20 浏览: 89
在MATLAB中,如果你想找出两个cell数组(类似于列表或数组的元素为字符串的数据结构)中相同的字符串,可以使用`isequal`函数配合循环来实现。以下是步骤:
1. 首先,创建两个cell数组,例如:
```matlab
cell1 = {'apple', 'banana', 'cherry'};
cell2 = {'orange', 'banana', 'grape'};
```
2. 然后,你可以遍历第一个cell数组,对于每个元素,检查它是否存在于第二个cell数组中:
```matlab
common_strings = {};
for i = 1:length(cell1)
if ismember(cell1{i}, cell2) % 使用ismember查找相同的元素
common_strings{end+1} = cell1{i}; % 如果找到,添加到结果数组
end
end
```
3. 最后,`common_strings`将包含两个cell数组中相同的字符串。如果没有任何共享的元素,`common_strings`将会是一个空cell。
如果你想要更简洁的方式,可以使用` intersect `函数结合` strcmp `进行比较:
```matlab
common_strings = intersect({cell1{:}}, {cell2{:}});
```
这将直接返回两个cell数组共有字符串的结果。
相关问题
matlab将表格中某一列不同的字符串分类换成其他的字符串
你可以使用 `replace` 函数来实现这个功能。假设你想将表格 `table` 中名为 `column1` 的列中的字符串分类进行替换,可以按照以下步骤进行操作:
1. 首先确定需要替换的字符串及其对应的新字符串。假设有字符串 "a", "b", "c" 需要替换成 "x",字符串 "d", "e", "f" 需要替换成 "y",则可以定义两个 cell 数组:
```
oldStrings = {'a', 'b', 'c', 'd', 'e', 'f'};
newStrings = {'x', 'x', 'x', 'y', 'y', 'y'};
```
2. 然后使用 `ismember` 函数找出需要替换的字符串在表格中的位置:
```
idx = ismember(table.column1, oldStrings);
```
3. 最后使用 `replace` 函数进行替换:
```
table.column1(idx) = newStrings{find(idx)};
```
完整的代码如下:
```
oldStrings = {'a', 'b', 'c', 'd', 'e', 'f'};
newStrings = {'x', 'x', 'x', 'y', 'y', 'y'};
idx = ismember(table.column1, oldStrings);
table.column1(idx) = newStrings{find(idx)};
```
阅读全文
相关推荐
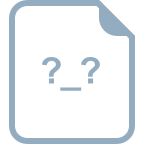
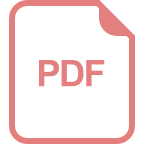
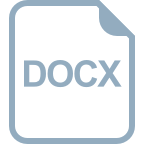
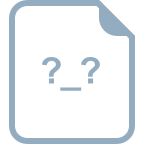
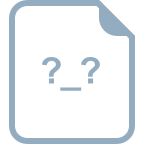
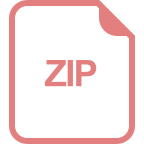
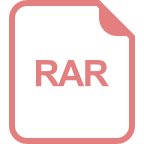
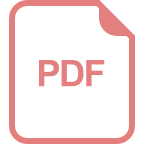
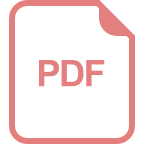
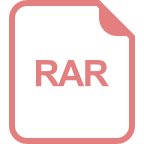
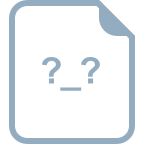
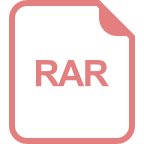
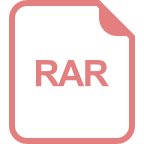
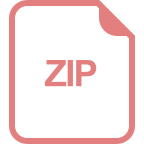
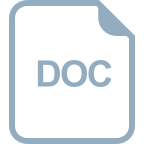
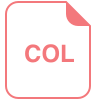
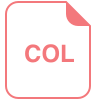
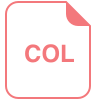
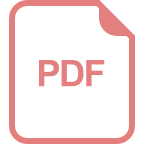