rust通过pyo3调用python 类方法
时间: 2023-09-06 18:08:21 浏览: 72
可以通过使用`pyo3`库来将`Python`类方法导出到`Rust`,然后在`Rust`中调用这些方法。以下是一个例子:
```python
# example.py
class MyClass:
def __init__(self, name):
self.name = name
def greet(self):
print(f"Hello, {self.name}!")
```
```rust
// main.rs
use pyo3::prelude::*;
use pyo3::wrap_pyfunction;
#[pyclass]
struct MyClass {
name: String,
}
#[pymethods]
impl MyClass {
#[new]
fn new(name: String) -> Self {
Self { name }
}
fn greet(&self) -> PyResult<()> {
Python::with_gil(|py| {
let gil = pyo3::Python::acquire_gil();
let py = gil.python();
let locals = [("self", pyo3::PyObject::from(self))].into_py_dict(py);
py.run("self.greet()", None, Some(&locals))?;
Ok(())
})
}
}
#[pyfunction]
fn create_my_class(name: String) -> PyResult<MyClass> {
Ok(MyClass::new(name))
}
#[pymodule]
fn example(_py: Python, m: &PyModule) -> PyResult<()> {
m.add_class::<MyClass>()?;
m.add_wrapped(wrap_pyfunction!(create_my_class))?;
Ok(())
}
fn main() -> PyResult<()> {
Python::with_gil(|py| {
let example_module = PyModule::new(py, "example")?;
example_module.add_class::<MyClass>()?;
example_module.add_wrapped(wrap_pyfunction!(create_my_class))?;
let locals = [("example", example_module)].into_py_dict(py);
py.run("import example", None, Some(&locals))?;
let my_class = example_module
.call("create_my_class", ("World",), None)?
.extract::<MyClass>()?;
my_class.greet()?;
Ok(())
})
}
```
这个例子中,我们使用`pyo3`库将`Python`类`MyClass`导出到`Rust`中。在`Rust`代码中,我们可以通过调用`MyClass`的`greet`方法来执行`Python`类中的`greet`方法。我们还编写了一个`Rust`函数`create_my_class`,用于创建`MyClass`实例并将其返回到`Python`。最后,我们在`Rust`中调用`Python`代码来创建`MyClass`实例,然后调用`greet`方法来打印出“Hello, World!”的消息。
相关推荐
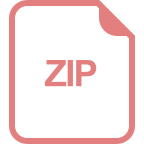














