用单链表为某个单位建立一个员工通讯录管理系统,可以方便查询每一个员工的手机号、及电子邮箱。其功能包括通讯录链表的建立、员工通讯信息的查询、修改、插入与删除、以及整个通讯录表的输出。 操作分为查询、修改、添加、删除、打印 1. 查询:输入员工姓名,输出员工信息,若员工不存在,输出“NOT FOUND!” 2. 修改:输入员工姓名、要修改的属性,修改信息。姓名为name、电话为tel、邮箱为mail。 3. 添加:输入员工编号、姓名、电话号码、邮箱。 4. 删除:输入员工姓名,将其从通讯录中删除。 5. 打印:输出通讯录。 代码
时间: 2023-07-10 18:05:13 浏览: 77
以下是一个简单的员工通讯录管理系统,使用了单链表的数据结构:
```python
class Employee:
def __init__(self, number, name, phone, email):
self.number = number
self.name = name
self.phone = phone
self.email = email
self.next = None
class AddressBook:
def __init__(self):
self.head = None
def add_employee(self, number, name, phone, email):
new_employee = Employee(number, name, phone, email)
if self.head is None:
self.head = new_employee
else:
current = self.head
while current.next is not None:
current = current.next
current.next = new_employee
def find_employee(self, name):
current = self.head
while current is not None:
if current.name == name:
return current
current = current.next
print("NOT FOUND!")
return None
def update_employee(self, name, attribute, value):
employee = self.find_employee(name)
if employee is not None:
if attribute == "name":
employee.name = value
elif attribute == "tel":
employee.phone = value
elif attribute == "mail":
employee.email = value
def delete_employee(self, name):
if self.head is None:
return
if self.head.name == name:
self.head = self.head.next
return
current = self.head
while current.next is not None:
if current.next.name == name:
current.next = current.next.next
return
current = current.next
def print_address_book(self):
current = self.head
while current is not None:
print("Number: {}, Name: {}, Phone: {}, Email: {}".format(current.number, current.name, current.phone, current.email))
current = current.next
```
使用方法:
```python
address_book = AddressBook()
# 添加员工
address_book.add_employee(1, "Alice", "1234567890", "alice@example.com")
address_book.add_employee(2, "Bob", "2345678901", "bob@example.com")
address_book.add_employee(3, "Charlie", "3456789012", "charlie@example.com")
# 查询员工
employee = address_book.find_employee("Alice")
if employee is not None:
print("Number: {}, Name: {}, Phone: {}, Email: {}".format(employee.number, employee.name, employee.phone, employee.email))
# 修改员工信息
address_book.update_employee("Bob", "tel", "3456789123")
# 删除员工
address_book.delete_employee("Charlie")
# 输出通讯录
address_book.print_address_book()
```
阅读全文
相关推荐
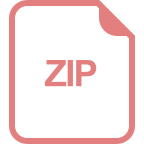
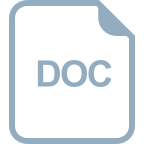


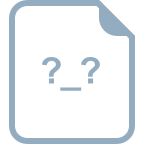
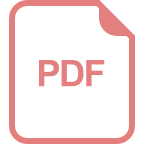
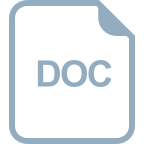
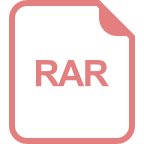
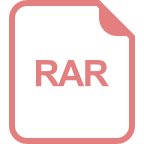