Linux C 下http服务器解析表单网页中以GET、POST方式上传的数据
时间: 2024-02-18 12:00:51 浏览: 187
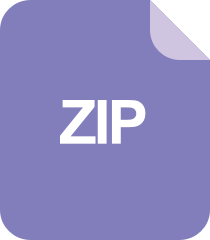
C语言开发Linux下web服务器(支持GET/POST,SSL,目录显示等)
在 Linux C 下开发 HTTP 服务器时,可以使用开源的第三方库来解析 HTTP 请求,例如 `libcurl`、`libmicrohttpd` 等等。这些库提供了方便的接口来解析 HTTP 请求,包括解析 URL、解析 POST 数据等等。
以下是使用 `libmicrohttpd` 库来解析 HTTP 请求的示例代码:
```c
#include <microhttpd.h>
static int handle_request(void *cls, struct MHD_Connection *connection,
const char *url, const char *method,
const char *version, const char *upload_data,
size_t *upload_data_size, void **con_cls)
{
// 解析 URL 和方法
if (strcmp(method, MHD_HTTP_METHOD_GET) == 0) {
// 处理 GET 请求
const char *name = MHD_lookup_connection_value(connection, MHD_GET_ARGUMENT_KIND, "name");
const char *age = MHD_lookup_connection_value(connection, MHD_GET_ARGUMENT_KIND, "age");
// ...
} else if (strcmp(method, MHD_HTTP_METHOD_POST) == 0) {
// 处理 POST 请求
// 获取 POST 数据
char post_data[1024];
size_t post_data_size = MHD_get_connection_values(connection, MHD_POSTDATA_KIND, post_data, sizeof(post_data));
// 解析 POST 数据
const char *name = NULL;
const char *age = NULL;
char *pos = post_data;
while (pos < post_data + post_data_size) {
char *key = pos;
char *value = strchr(pos, '=');
if (value == NULL) {
break;
}
*value++ = '\0';
char *end = strchr(value, '&');
if (end != NULL) {
*end++ = '\0';
} else {
end = post_data + post_data_size;
}
if (strcmp(key, "name") == 0) {
name = value;
} else if (strcmp(key, "age") == 0) {
age = value;
}
pos = end;
}
// ...
}
// 返回响应
const char *response = "Hello, World!";
struct MHD_Response *resp = MHD_create_response_from_buffer(strlen(response), (void *) response, MHD_RESPMEM_PERSISTENT);
MHD_add_response_header(resp, "Content-Type", "text/plain");
int ret = MHD_queue_response(connection, MHD_HTTP_OK, resp);
MHD_destroy_response(resp);
return ret;
}
int main()
{
struct MHD_Daemon *daemon = MHD_start_daemon(MHD_USE_SELECT_INTERNALLY, 8080, NULL, NULL,
&handle_request, NULL, MHD_OPTION_END);
if (daemon == NULL) {
printf("Failed to start daemon\n");
return 1;
}
getchar();
MHD_stop_daemon(daemon);
return 0;
}
```
在以上示例代码中,`handle_request` 函数用于处理 HTTP 请求。在函数中,首先根据请求的方法来解析 URL 或 POST 数据。对于 GET 请求,可以使用 `MHD_lookup_connection_value` 函数来解析 URL 参数;对于 POST 请求,可以使用 `MHD_get_connection_values` 函数来获取 POST 数据,然后自行解析 POST 数据。
需要注意的是,在解析 POST 数据时,需要手动解析 POST 数据的格式。通常情况下,POST 数据的格式为 `key1=value1&key2=value2&...`,需要根据这个格式来解析 POST 数据。
最后,需要根据请求返回相应的响应。在示例代码中,返回了一个简单的响应 "Hello, World!"。
阅读全文
相关推荐
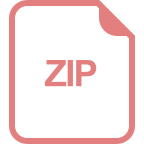
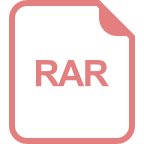















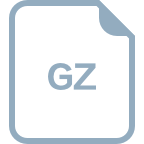