autopoi excel模板导出 java实例
时间: 2023-08-01 07:09:41 浏览: 164
好的,这里是一个基于 Autopoi 的 Excel 模板导出 Java 实例。
首先,你需要在你的项目中添加 Autopoi 的依赖。如果你使用 Maven,可以在 `pom.xml` 文件中添加以下代码:
```xml
<dependency>
<groupId>cn.afterturn</groupId>
<artifactId>autopoio</artifactId>
<version>2.1.0</version>
</dependency>
```
然后,你需要准备好 Excel 模板文件,这个文件中可以包含一些占位符,例如 `${name}`。
接下来,你需要创建一个 Java 类,用于解析模板文件并填充数据。这个类可以继承 `AbstractExcelView` 类,并实现 `buildExcelDocument()` 方法。以下是一个简单的例子:
```java
public class MyExcelView extends AbstractExcelView {
@Override
protected void buildExcelDocument(Map<String, Object> model, Workbook workbook, HttpServletRequest request,
HttpServletResponse response) throws Exception {
// 获取数据
List<MyData> dataList = (List<MyData>) model.get("dataList");
// 加载模板文件
InputStream inputStream = getClass().getResourceAsStream("/templates/my_template.xlsx");
XSSFWorkbook wb = new XSSFWorkbook(inputStream);
// 获取模板中的第一个工作表
XSSFSheet sheet = wb.getSheetAt(0);
// 填充数据
int rowIndex = 1;
for (MyData data : dataList) {
XSSFRow row = sheet.createRow(rowIndex++);
row.createCell(0).setCellValue(data.getName());
row.createCell(1).setCellValue(data.getValue());
}
// 输出 Excel 文件
response.setContentType("application/vnd.ms-excel");
String fileName = "my_excel.xlsx";
response.setHeader("Content-disposition", "attachment;filename=" + URLEncoder.encode(fileName, "UTF-8"));
OutputStream outputStream = response.getOutputStream();
wb.write(outputStream);
outputStream.flush();
outputStream.close();
}
}
```
在这个例子中,我们假设模板文件名为 `my_template.xlsx`,并且包含两列数据:`name` 和 `value`。
最后,你需要在你的控制器中调用这个视图,例如:
```java
@GetMapping("/download")
public ModelAndView download() {
List<MyData> dataList = ...; // 获取数据
ModelAndView mav = new ModelAndView(new MyExcelView());
mav.addObject("dataList", dataList);
return mav;
}
```
现在,当你访问 `/download` 路径时,就会下载一个填充好数据的 Excel 文件。
阅读全文
相关推荐
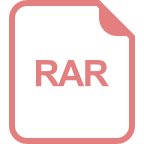
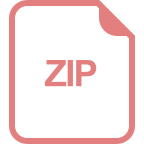
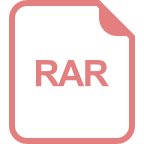
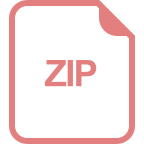
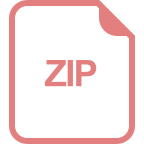
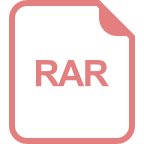
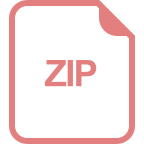
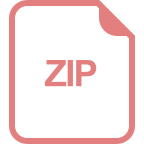
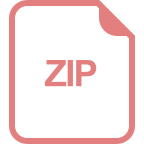
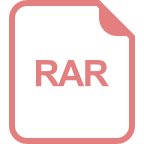
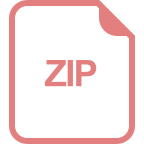