前端获取的接口数据是一个数组,每个元素有 id、type、username等怎么遍历数组,判断是否有type为4且username为特定值
时间: 2024-09-28 21:15:54 浏览: 9
在前端开发中,我们经常需要处理从接口获取的数据,其中数组是常见的数据格式。假设你获取的数组包含多个元素,每个元素都有 `id`、`type` 和 `username` 等属性。现在我们需要遍历这个数组,判断是否有 `type` 为4且 `username` 为特定值的元素。
你可以使用JavaScript中的多种方法来实现这一目标,比如 `forEach`、`for...of`、`find` 等等。以下是几种常见的实现方式:
### 1. 使用 `forEach` 方法
```javascript
const data = [
{ id: 1, type: 3, username: 'Alice' },
{ id: 2, type: 4, username: 'Bob' },
{ id: 3, type: 2, username: 'Charlie' }
];
let specificUsername = 'Bob';
let found = false;
data.forEach(item => {
if (item.type === 4 && item.username === specificUsername) {
found = true;
}
});
console.log(found); // 输出: true
```
### 2. 使用 `for...of` 循环
```javascript
const data = [
{ id: 1, type: 3, username: 'Alice' },
{ id: 2, type: 4, username: 'Bob' },
{ id: 3, type: 2, username: 'Charlie' }
];
let specificUsername = 'Bob';
let found = false;
for (const item of data) {
if (item.type === 4 && item.username === specificUsername) {
found = true;
break;
}
}
console.log(found); // 输出: true
```
### 3. 使用 `find` 方法(推荐)
```javascript
const data = [
{ id: 1, type: 3, username: 'Alice' },
{ id: 2, type: 4, username: 'Bob' },
{ id: 3, type: 2, username: 'Charlie' }
];
let specificUsername = 'Bob';
let foundItem = data.find(item => item.type === 4 && item.username === specificUsername);
console.log(!!foundItem); // 输出: true
```
### 4. 使用 `some` 方法
```javascript
const data = [
{ id: 1, type: 3, username: 'Alice' },
{ id: 2, type: 4, username: 'Bob' },
{ id: 3, type: 2, username: 'Charlie' }
];
let specificUsername = 'Bob';
let found = data.some(item => item.type === 4 && item.username === specificUsername);
console.log(found); // 输出: true
```
这几种方法都可以有效地遍历数组并判断是否存在满足条件的元素。选择哪种方法可以根据你的具体需求和个人习惯。
相关推荐
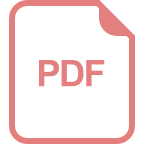
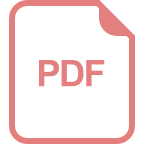
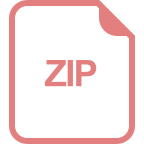









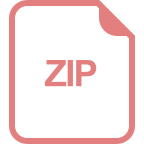
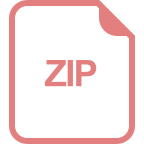
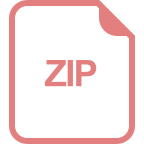
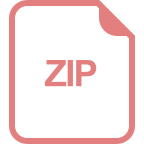