用C语言在C51硬件平台上实现串口的不定长中断接收功能,并且支持超时判断
时间: 2024-03-05 08:53:24 浏览: 187
实现串口的不定长中断接收功能并支持超时判断,需要在上面的代码基础上增加超时计数器和定时器中断。以下是一份简单的C语言代码示例:
```c
#include <reg51.h>
#define UART_BAUD_RATE 9600
#define UART_BUFFER_SIZE 64
#define UART_TIMEOUT_MS 1000
unsigned char uart_rx_buffer[UART_BUFFER_SIZE];
unsigned char uart_rx_buffer_head = 0;
unsigned char uart_rx_buffer_tail = 0;
unsigned int uart_timeout_counter = 0;
void uart_init()
{
/* 配置波特率 */
TH1 = 256 - (11059200UL / 12 / UART_BAUD_RATE);
TL1 = TH1;
/* 配置串口 */
SCON = 0x50;
TMOD &= 0x0F;
TMOD |= 0x20;
TR1 = 1;
/* 配置中断 */
ES = 1;
EA = 1;
/* 配置定时器 */
TMOD &= 0xF0;
TMOD |= 0x01;
TH0 = 0x3C;
TL0 = 0xAF;
ET0 = 1;
TR0 = 1;
}
void uart_isr() interrupt 4
{
if (RI) {
RI = 0;
/* 接收到数据,存入缓存 */
uart_rx_buffer[uart_rx_buffer_head] = SBUF;
uart_rx_buffer_head = (uart_rx_buffer_head + 1) % UART_BUFFER_SIZE;
/* 重置超时计数器 */
uart_timeout_counter = 0;
}
}
void timer0_isr() interrupt 1
{
/* 定时器中断,增加超时计数器 */
TH0 = 0x3C;
TL0 = 0xAF;
uart_timeout_counter++;
}
unsigned char uart_read(unsigned int timeout_ms)
{
unsigned char data;
unsigned int timeout_counter = 0;
/* 等待直到收到数据或超时 */
while (uart_rx_buffer_head == uart_rx_buffer_tail && timeout_counter < timeout_ms) {
/* 延时1ms */
delay_ms(1);
timeout_counter++;
}
/* 如果超时,返回0 */
if (timeout_counter >= timeout_ms) {
return 0;
}
/* 从缓存中读取数据 */
data = uart_rx_buffer[uart_rx_buffer_tail];
uart_rx_buffer_tail = (uart_rx_buffer_tail + 1) % UART_BUFFER_SIZE;
return data;
}
void main()
{
unsigned char data;
uart_init();
while (1) {
/* 从串口缓存中读取数据 */
data = uart_read(UART_TIMEOUT_MS);
if (data) {
/* 处理接收到的数据 */
// ...
}
}
}
```
在上面的代码中,增加了超时计数器和定时器中断服务函数。读取串口数据时,使用循环等待的方式直到接收到数据或超时。如果超时,则返回0。每次接收到数据时,重置超时计数器。定时器中断每1ms触发一次,用于增加超时计数器。这样,即使串口接收到的数据长度不确定,也可以实现中断接收并支持超时判断。
阅读全文
相关推荐
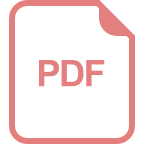
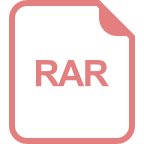
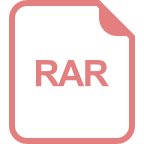
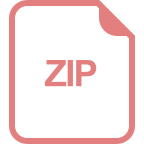
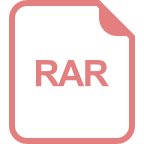
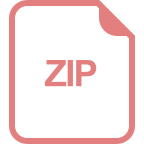
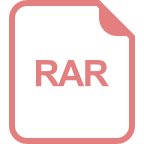
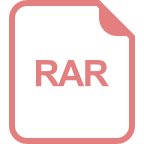
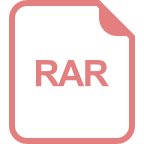
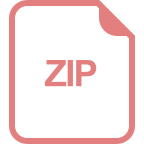
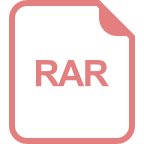
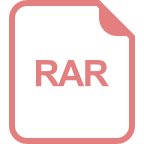
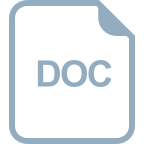
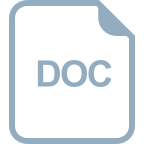
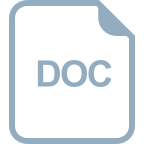
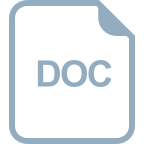
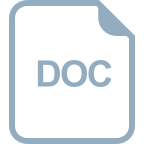