java贝塞尔曲线与直线的交点
时间: 2023-09-03 18:16:27 浏览: 180
要确定Java中贝塞尔曲线和直线的交点,您可以使用Java 2D API和Java几何类来实现。下面是一个处理贝塞尔曲线和直线交点的例子:
首先,您需要创建一个Java 2D对象,如下所示:
```
Path2D.Double curve = new Path2D.Double();
curve.moveTo(0, 0);
curve.curveTo(25, 100, 75, -100, 100, 0);
```
这将创建一个三次贝塞尔曲线,其中起点是(0,0),控制点1是(25,100),控制点2是(75,-100),终点是(100,0)。
接下来,您需要创建一条线段,如下所示:
```
Line2D.Double line = new Line2D.Double(0, 50, 100, 50);
```
这将创建一个从(0,50)到(100,50)的直线。
现在,您可以使用Java几何类的intersect方法来找到贝塞尔曲线和线段的交点:
```
PathIterator pi = curve.getPathIterator(null);
double[] coords = new double[6];
double prevX = 0, prevY = 0;
while (!pi.isDone()) {
int segType = pi.currentSegment(coords);
switch (segType) {
case PathIterator.SEG_MOVETO:
prevX = coords[0];
prevY = coords[1];
break;
case PathIterator.SEG_LINETO:
Line2D.Double segLine = new Line2D.Double(prevX, prevY, coords[0], coords[1]);
Point2D.Double intersection = getIntersection(line, segLine);
if (intersection != null) {
// Do something with the intersection point
}
prevX = coords[0];
prevY = coords[1];
break;
case PathIterator.SEG_QUADTO:
// TODO: Handle quadratic curve segment
break;
case PathIterator.SEG_CUBICTO:
CubicCurve2D.Double cubicCurve = new CubicCurve2D.Double(prevX, prevY, coords[0], coords[1], coords[2], coords[3], coords[4], coords[5]);
List<Point2D.Double> intersections = getIntersections(line, cubicCurve);
for (Point2D.Double intersection : intersections) {
// Do something with the intersection point
}
prevX = coords[4];
prevY = coords[5];
break;
case PathIterator.SEG_CLOSE:
break;
}
pi.next();
}
```
在这里,我们使用getPathIterator方法来遍历贝塞尔曲线的所有线段。对于每个线段,我们使用Java几何类的Line2D.Double来创建一个线段对象。然后,我们使用自定义的getIntersection方法来找到贝塞尔曲线和线段的交点。如果找到交点,则可以对其进行处理。
对于三次贝塞尔曲线,我们可以使用Java几何类的CubicCurve2D.Double创建一个曲线对象。然后,我们使用自定义的getIntersections方法来找到贝塞尔曲线和线段的交点列表。如果找到交点,则可以对其进行处理。
以下是getIntersection方法的示例实现:
```
public static Point2D.Double getIntersection(Line2D.Double line1, Line2D.Double line2) {
double x1 = line1.x1, y1 = line1.y1;
double x2 = line1.x2, y2 = line1.y2;
double x3 = line2.x1, y3 = line2.y1;
double x4 = line2.x2, y4 = line2.y2;
double denom = (y4-y3)*(x2-x1)-(x4-x3)*(y2-y1);
if (denom == 0) {
return null; // Lines are parallel
}
double ua = ((x4-x3)*(y1-y3)-(y4-y3)*(x1-x3))/denom;
double ub = ((x2-x1)*(y1-y3)-(y2-y1)*(x1-x3))/denom;
if (ua >= 0 && ua <= 1 && ub >= 0 && ub <= 1) {
double x = x1 + ua*(x2-x1);
double y = y1 + ua*(y2-y1);
return new Point2D.Double(x, y);
}
return null; // Intersection point is outside of line segments
}
```
该方法使用两条线段的坐标来计算它们的交点。如果找到交点,则返回一个Point2D.Double对象,否则返回null。
以下是getIntersections方法的示例实现:
```
public static List<Point2D.Double> getIntersections(Line2D.Double line, CubicCurve2D.Double curve) {
List<Point2D.Double> intersections = new ArrayList<Point2D.Double>();
for (double t = 0; t <= 1; t += 0.01) {
Point2D.Double point = new Point2D.Double(curve.getX(t), curve.getY(t));
if (line.ptSegDist(point) == 0) {
intersections.add(point);
}
}
return intersections;
}
```
该方法使用线段和贝塞尔曲线的坐标来计算它们的交点。我们使用一个循环来遍历贝塞尔曲线上的点,并检查它们是否与线段相交。如果找到交点,则将其添加到一个列表中。
希望这可以帮助您找到Java中贝塞尔曲线和直线的交点。
阅读全文
相关推荐
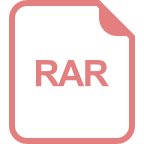
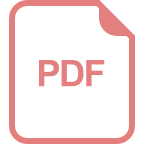
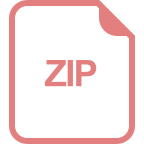
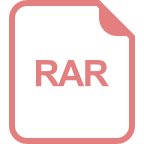
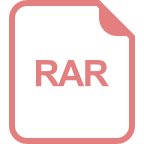
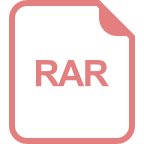
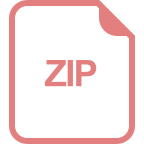
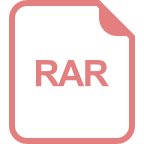
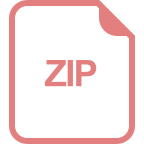
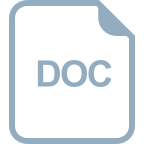
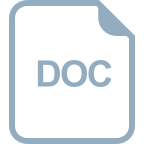

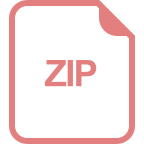
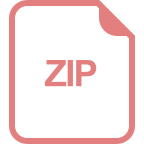
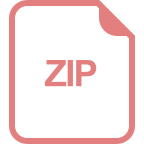
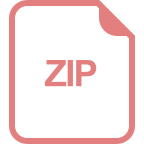