vue2+element-ui评论展示功能
时间: 2023-08-26 17:05:08 浏览: 59
实现评论展示功能可以分为以下几个步骤:
1. 创建评论数据结构
首先需要定义一个评论的数据结构,例如:
```
{
id: 1, // 评论ID
username: '张三', // 评论人姓名
content: '这是一条评论', // 评论内容
createTime: '2021-09-01 10:00:00', // 评论时间
replies: [
// 子评论列表
{
id: 2,
username: '李四',
content: '回复张三的评论',
createTime: '2021-09-01 10:05:00'
},
// ...
]
}
```
2. 展示评论列表
使用 `el-table` 组件展示评论列表,例如:
```
<el-table :data="comments">
<el-table-column label="评论人" prop="username"></el-table-column>
<el-table-column label="评论内容" prop="content"></el-table-column>
<el-table-column label="评论时间" prop="createTime"></el-table-column>
</el-table>
```
其中,`comments` 是包含所有评论的数组。
3. 实现回复功能
在每条评论下方添加一个回复框,用于回复该评论。例如:
```
<el-table :data="comments">
<el-table-column label="评论人" prop="username"></el-table-column>
<el-table-column label="评论内容" prop="content"></el-table-column>
<el-table-column label="评论时间" prop="createTime"></el-table-column>
<el-table-column label="操作">
<template slot-scope="scope">
<el-button @click="reply(scope.row)">回复</el-button>
<el-dialog :visible.sync="scope.row.showReplyDialog">
<el-input v-model="scope.row.replyContent"></el-input>
<el-button @click="submitReply(scope.row)">提交</el-button>
</el-dialog>
</template>
</el-table-column>
</el-table>
```
其中,`reply` 方法用于打开回复框,`submitReply` 方法用于提交回复内容。需要在评论数据结构中添加 `showReplyDialog` 和 `replyContent` 字段,分别表示回复框的显示状态和回复内容。
4. 实现子评论展示
使用 `el-table` 和递归组件展示子评论列表,例如:
```
<template>
<div>
<el-table :data="comments">
<el-table-column label="评论人" prop="username"></el-table-column>
<el-table-column label="评论内容" prop="content"></el-table-column>
<el-table-column label="评论时间" prop="createTime"></el-table-column>
<el-table-column label="操作">
<template slot-scope="scope">
<el-button @click="reply(scope.row)">回复</el-button>
<el-dialog :visible.sync="scope.row.showReplyDialog">
<el-input v-model="scope.row.replyContent"></el-input>
<el-button @click="submitReply(scope.row)">提交</el-button>
</el-dialog>
</template>
</el-table-column>
</el-table>
<div v-for="comment in comments" :key="comment.id">
<comment :data="comment"></comment>
</div>
</div>
</template>
<script>
export default {
components: {
Comment: {
props: {
data: {
type: Object,
required: true
}
},
template: `
<div>
<el-table :data="data.replies">
<el-table-column label="评论人" prop="username"></el-table-column>
<el-table-column label="评论内容" prop="content"></el-table-column>
<el-table-column label="评论时间" prop="createTime"></el-table-column>
</el-table>
<div v-for="reply in data.replies" :key="reply.id">
<comment :data="reply"></comment>
</div>
</div>
`
}
},
data() {
return {
comments: []
}
}
}
</script>
```
其中,`Comment` 组件用于递归展示子评论列表。需要在评论数据结构中添加 `replies` 字段,表示子评论列表。
相关推荐
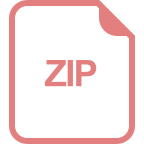
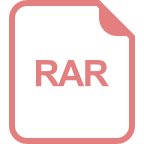
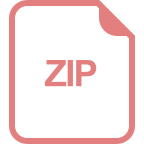















