用c语言编写数组最大值函数
时间: 2024-09-14 08:07:41 浏览: 15
在C语言中编写一个求取数组最大值的函数,通常需要以下几个步骤:
1. 定义函数,确定函数的返回类型、名称和参数。例如,返回类型可以是int,函数名为`findMax`,参数包括数组名和数组中元素的个数。
2. 在函数内部初始化一个变量来存储当前遇到的最大值,通常将数组的第一个元素作为初始最大值。
3. 遍历数组中的每个元素,使用循环结构(例如for循环或while循环)。
4. 在每次循环中,将当前元素与当前已知的最大值进行比较,如果当前元素更大,则更新最大值变量。
5. 遍历完成后,返回最大值变量。
下面是一个简单的示例代码:
```c
#include <stdio.h>
// 函数声明
int findMax(int arr[], int n);
int main() {
int array[] = {3, 5, 7, 2, 9, 10};
int max = findMax(array, sizeof(array)/sizeof(array[0]));
printf("数组的最大值是:%d\n", max);
return 0;
}
// 函数定义
int findMax(int arr[], int n) {
int max = arr[0]; // 假设第一个元素为最大值
for (int i = 1; i < n; i++) {
if (arr[i] > max) {
max = arr[i]; // 更新最大值
}
}
return max; // 返回最大值
}
```
相关推荐
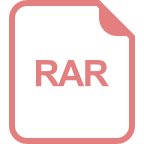
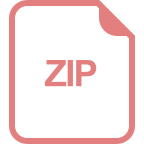
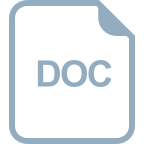
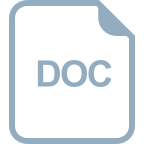
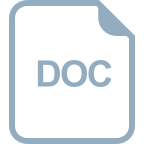
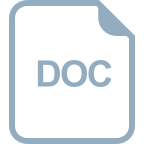
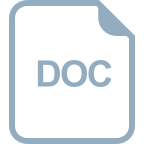
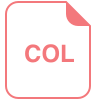
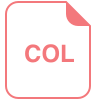
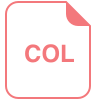
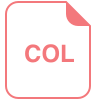
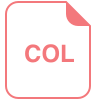




