How to add plotly to pyqt5
时间: 2024-10-04 13:04:48 浏览: 135
To add Plotly to PyQt5, you need to follow these steps:
1. **安装依赖库**:
- First, ensure you have Python and pip installed. If not, visit https://www.python.org/downloads/ for the latest Python installer.
- Install `plotly` library using pip by running `pip install plotly`.
- If you want interactive features with Qt, you'll also need `pyqtgraph`, which is a plotting library built on top of PyQt5. Install it with `pip install pyqtgraph`.
2. **导入所需模块**:
In your Python script that uses PyQt5, import the necessary modules:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow
from plotly.graph_objs import Figure
from plotly.offline import plot
from pyqtgraph.Qt import QtGui, QtCore
```
3. **创建Qt应用**:
Initialize your PyQt5 application and main window:
```python
app = QApplication([])
MainWindow = QMainWindow()
```
4. **绘制Plotly图形**:
使用PyQtGraph's `PlotWidget`或`PlotItem`来显示Plotly图形。例如, 创建一个简单的 line chart:
```python
fig = Figure(data=[dict(type='scatter', x=[1, 2, 3], y=[4, 1, 2])])
graph_widget = PlotWidget(figure=fig)
graph_widget.show()
```
5. **渲染Plotly在窗口上**:
You can then connect this widget to your PyQt window and display it:
```python
MainWindow.setCentralWidget(graph_widget)
MainWindow.show()
```
6. **事件循环**:
Start the event loop to run the GUI:
```python
app.exec_()
```
**相关问题--:**
1. 如何在PyQt5中嵌入静态Plotly图表而不是实时更新的?
2. 如果我想让Plotly图形响应用户交互,应该如何集成到Qt界面中?
3. 如果我在运行过程中遇到关于版本兼容性的错误,应该怎么做?
阅读全文
相关推荐


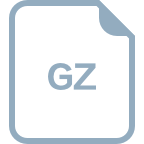












