error: argument of type "int" is incompatible with parameter of type "const char *"
时间: 2024-11-22 10:37:38 浏览: 37
这个编译错误提示意味着你在尝试将一个整型变量(int)作为常量字符指针(const char*)传递给某个函数或方法。在C和C++中,`const char *`通常用于表示字符串,而`int`是一个数值类型。函数期望的是指向字符串的指针,而不是一个整数。
举个例子,如果你有以下代码:
```c
void printString(const char* str) {
// 函数用来打印字符串
printf("%s", str);
}
int main() {
int num = 123;
printString(num); // 错误,num不是一个有效的字符串指针
}
```
在这个例子中,`printString()`函数期望一个字符串地址,但是你却给了它一个整数`num`的地址,这就会导致编译错误。
解决这个问题的方法是将`int`转换成`const char *`(字符串),如果是数字的话,需要先将其转换为字符串再传递,或者修改函数接受`int`作为参数并自行转换。例如:
```c
void printIntAsStr(int num) {
printf("%d", num);
}
int main() {
int num = 123;
printIntAsStr(num); // 正确,num会被当作整数打印
}
```
相关问题
error: argument of type "int *" is incompatible with parameter of type "int **"
This error occurs when a function is expecting a pointer to a pointer (e.g. int **), but a pointer to a single integer (e.g. int *) is passed as an argument.
For example, consider the following code:
```c
void foo(int **p) {
// do something with p
}
int main() {
int x = 5;
foo(&x); // ERROR: argument of type "int *" is incompatible with parameter of type "int **"
return 0;
}
```
In this example, the function `foo` expects a pointer to a pointer to an integer, but we are passing a pointer to a single integer `x`. To fix this error, we need to pass the address of a pointer to an integer, like this:
```c
void foo(int **p) {
// do something with p
}
int main() {
int x = 5;
int *ptr = &x;
foo(&ptr); // OK
return 0;
}
```
Here, we create a pointer `ptr` that points to `x`, and then pass the address of `ptr` to `foo`. Now, `foo` can access `x` through the pointer `ptr`.
上面代码出错main.c(72): error: #167: argument of type "int" is incompatible with parameter of type "const char *"
这个错误表明你在使用`printf`函数时传入了一个整型`int`作为字符串格式说明符,而`printf`需要的是指向常量字符数组的指针(即`const char *`)。在C语言中,`atof`函数用于把字符串转换成浮点数,它的返回类型是`double`,所以这里的`printf("%.1f", original_value);`会隐式地将`double`类型的`original_value`转换成整型,然后再传递给`printf`。
修复这个错误,你需要直接获取`printf`的返回值,而不是将其赋值给`rounded_value`:
```c
#include <stdio.h>
#include <stdlib.h> // 需要包含stdlib.h才能使用atoi
double original_value = 20.26546;
char formatted_str[10]; // 创建一个临时字符数组存放格式化结果
sprintf(formatted_str, "%.1f", original_value); // 使用sprintf来保存格式化的字符串
double rounded_value = atof(formatted_str);
if (rounded_value == -1) {
printf("转换失败,原始值可能不是有效浮点数\n");
} else {
printf("保留一位小数后的值是: %.1f\n", rounded_value);
}
阅读全文
相关推荐
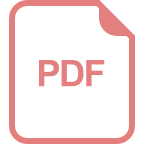
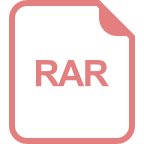
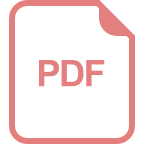













