qt c++ openssl RSA 加签 和验签
时间: 2024-10-19 18:17:15 浏览: 44
在Qt C++中使用OpenSSL进行RSA加签(Signing)和验签(Verification),首先需要包含相关的头文件并初始化OpenSSL库。以下是一个简化的步骤说明:
**1. 包含必要的头文件**:
```cpp
#include <QFile>
#include <QByteArray>
#include <openssl/pem.h>
#include <openssl/rsa.h>
```
**2. 加载私钥(用于签名)**:
```cpp
RSA* rsaPrivateKey = nullptr;
QFile privateKeyFile("private_key.pem");
if (!privateKeyFile.open(QIODevice::ReadOnly)) {
// 处理错误
}
char* pemData = new char[privateKeyFile.size()];
privateKeyFile.read(pemData, privateKeyFile.size());
pem_data = reinterpret_cast<char*>(PEM_read_PrivateKey(privateKeyFile.data(), &rsaPrivateKey, NULL, NULL));
```
**3. 加密消息并生成数字签名(Signature)**:
```cpp
std::string message = "Your message to sign";
unsigned char hash[SHA256_DIGEST_LENGTH];
CryptoPP::SHA256(message.begin(), message.length(), hash);
QRsaSignature signer(*rsaPrivateKey, CryptoPP::RSASSA_PKCS1_v1_5());
QByteArray signature = signer.sign(QByteArray((const char*)hash, SHA256_DIGEST_LENGTH));
```
**4. 验证签名**:
```cpp
RSA* rsaPublicKey; // 加载公钥
// ...
QRsaVerifier verifier(*rsaPublicKey, CryptoPP::RSASSA_PKCS1_v1_5());
bool isValid = verifier.verify(signature.data(), signature.size(), (const unsigned char*)hash, SHA256_DIGEST_LENGTH);
```
**相关问题--**:
1. OpenSSL中如何保存和加载公钥证书?
2. 如果私钥丢失,如何验证已有的签名?
3. 如何处理签名过程中可能出现的错误或异常情况?
阅读全文
相关推荐
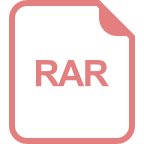
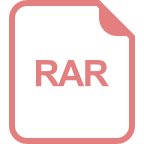
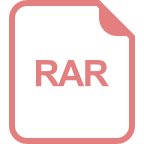
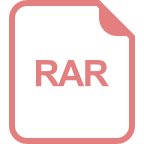
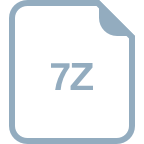
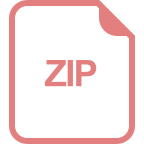
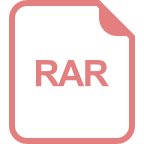
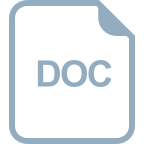
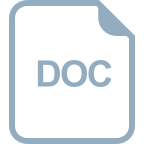
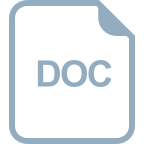
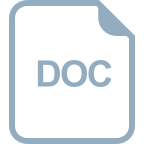
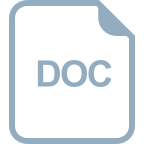
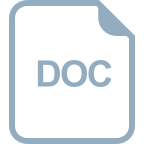
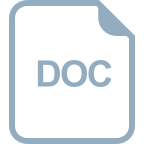





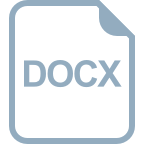