TypeError: Cannot read properties of undefined (reading 'toggleSelection
时间: 2024-01-24 15:16:05 浏览: 178
这个错误是由于尝试读取未定义对象的属性'toggleSelection'而引起的。通常,这种错误可以通过添加nextTick或setTimeout来解决。下面是一个示例代码:
```javascript
const multipleTableRef = ref() // 绑定在Table上的Ref
const toggleSelection = rows => {
rows.forEach(row => {
multipleTableRef.value!.toggleRowSelection(row, true)
})
}
onMounted(() => {
nextTick(() => {
toggleSelection(tableData.value)
})
})
```
在这个示例中,我们使用了Vue的`ref`函数来创建一个绑定在Table组件上的引用`multipleTableRef`。然后,我们定义了一个`toggleSelection`函数,它接受一个行数组作为参数,并使用`multipleTableRef`引用来调用`toggleRowSelection`方法来切换行的选择状态。最后,在组件的`onMounted`生命周期钩子中,我们使用`nextTick`函数来确保在Table组件完成页面渲染后再调用`toggleSelection`函数。
相关问题
TypeError: Cannot read properties of undefined (reading 'toggleSelection')
TypeError: Cannot read properties of undefined (reading 'toggleSelection') 是一个JavaScript中常见的运行时错误。当你尝试访问一个变量、对象属性或方法,但该变量或对象的值是 `undefined`(即没有定义或为 `null`),JavaScript就会抛出这个错误。`'toggleSelection'` 在这里看起来像是一个函数名,可能是你在尝试调用某个对象的方法,但那个对象在当前上下文中并没有初始化或者已经被修改为 `undefined`。
例如,假设你有一个这样的代码片段:
```javascript
let myElement = {}; // 这里 myElement 没有 toggleSelection 属性
myElement.toggleSelection(); // 这会导致 TypeError
```
为了解决这个问题,你需要检查以下几点:
1. 确保 `toggleSelection` 是 `myElement` 的正确属性或方法。
2. 在访问之前,检查 `myElement` 是否已经定义并赋值了。
3. 如果 `toggleSelection` 是可选的,考虑使用可选链操作 (`?.`) 或者默认参数来避免错误。
Cannot read properties of undefined (reading 'error') TypeError: Cannot read properties of undefined
这个错误通常是由于尝试访问未定义或空值的属性而导致的。例如,如果您尝试访问未定义的对象或变量的属性,则会出现此错误。
以下是一些可能导致此错误的原因:
1. 您尝试访问未定义或空值的对象或变量。
2. 您正在调用一个函数,但它返回了 undefined 或 null。
3. 您正在使用一个不存在的对象或变量。
4. 您正在使用一个已被删除或销毁的对象或变量。
如果您遇到此错误,请检查您的代码并确保您正在正确地处理对象和变量。确保您正在正确地初始化和设置所有变量和对象,并在需要时检查它们是否为 undefined 或 null。
阅读全文
相关推荐
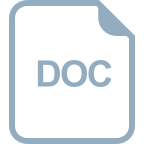
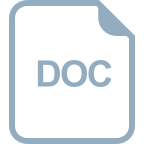
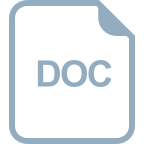













