在c语言中,如何在列表中寻找最大元素,并将该元素移动到列表头部?
时间: 2024-11-27 18:23:51 浏览: 8
在 C 语言中,要在链表中找到最大元素并将其移到头部,通常可以采用以下步骤:
1. 定义链表结构(如果尚未定义):
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
2. 初始化链表头指针和一个临时最大值变量:
```c
Node* head = NULL; // 链表头节点
int max_data = INT_MIN; // 初始化为整型最小值
```
3. 遍历链表:
```c
Node* current = head;
while (current != NULL) {
if (current->data > max_data) {
max_data = current->data; // 更新最大值
}
current = current->next;
}
```
4. 找到最大元素后,在遍历过程中更新最大元素的位置,使其指向原来的最大元素:
```c
Node* max_node = current;
current = current->next;
while (current != NULL && current->data == max_data) {
max_node = current;
current = current->next;
}
// 确保max_node仍然指向原位置
if (max_node != head && max_node->next != NULL) {
// 保存当前节点的下一个节点
Node* temp = max_node->next;
// 将最大元素移到头部
max_node->next = head;
head = max_node;
// 将原最大元素插入到链表前端
temp->next = head->next;
head = temp;
}
```
5. 最后,`head`现在指向了链表中的最大元素。
阅读全文
相关推荐
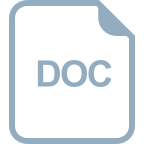
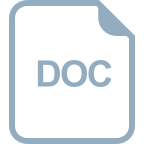
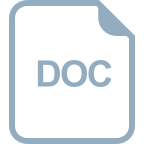
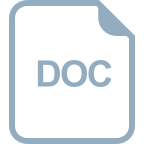
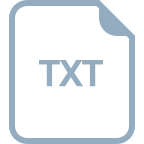
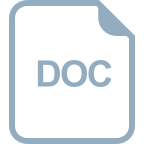
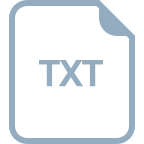
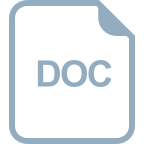
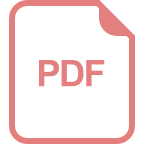
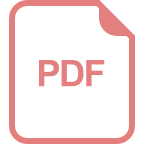
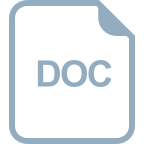
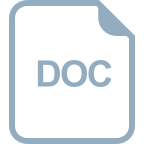
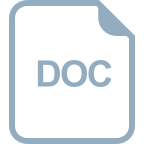
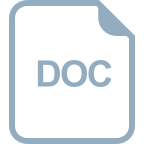
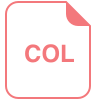
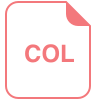
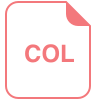
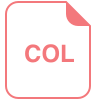
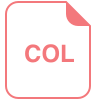