RestTemplate发起post请求
时间: 2023-10-12 14:05:56 浏览: 42
RestTemplate是Spring Framework提供的一个用于进行HTTP请求的类库。要使用RestTemplate发起POST请求,你可以按照以下步骤进行操作:
1. 首先,你需要创建一个RestTemplate对象,可以通过在Spring容器中进行注入或者直接实例化来获取一个RestTemplate实例。
2. 创建一个表示请求体的对象,可以是一个POJO对象或者是一个Map对象,根据具体的需求来决定。
3. 如果有需要,你可以设置请求头信息,例如设置Content-Type、Authorization等。
4. 调用RestTemplate对象的postForObject()方法来发送POST请求,并且接收响应结果。
下面是一个使用RestTemplate发起POST请求的示例代码:
```java
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
public class PostExample {
public static void main(String[] args) {
// 创建RestTemplate对象
RestTemplate restTemplate = new RestTemplate();
// 创建请求体对象
YourRequestObject requestObject = new YourRequestObject();
// 设置请求头信息
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
// 创建HttpEntity对象,用于封装请求体和请求头
HttpEntity<YourRequestObject> httpEntity = new HttpEntity<>(requestObject, headers);
// 发送POST请求,并接收响应结果
ResponseEntity<YourResponseObject> responseEntity = restTemplate.exchange(
"http://example.com/api/endpoint", // 请求URL
HttpMethod.POST, // 请求方法
httpEntity, // 请求体和请求头
YourResponseObject.class // 响应结果类型
);
// 获取响应结果
YourResponseObject responseObject = responseEntity.getBody();
// 处理响应结果
}
}
```
以上代码中的`YourRequestObject`和`YourResponseObject`分别表示请求体和响应结果的自定义对象,你需要根据实际情况进行替换。另外,根据你的需求可能需要进行异常处理、日志记录等其他操作。
相关推荐
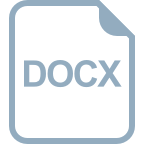














