帮我写一下FSK谱峰个数检测matlab代码
时间: 2023-06-16 11:06:03 浏览: 64
当使用FSK调制时,信号的频率可能会发生变化,因此检测这些频率峰并确定其数目非常重要。以下是一段MATLAB代码,用于检测FSK信号的谱峰个数:
```matlab
% 生成FSK信号
fs = 10000; % 采样率
f1 = 1000; % 第一个频率
f2 = 2000; % 第二个频率
t = 0:1/fs:1; % 时间
x = cos(2*pi*f1*t) + cos(2*pi*f2*t); % FSK信号
% 计算信号的功率谱密度
N = length(x);
xdft = fft(x);
xdft = xdft(1:N/2+1);
psdx = (1/(fs*N)) * abs(xdft).^2;
psdx(2:end-1) = 2*psdx(2:end-1);
freq = 0:fs/length(x):fs/2;
% 检测谱峰个数
[pks, locs] = findpeaks(psdx, freq);
num_peaks = length(pks);
disp(['谱峰个数:', num2str(num_peaks)]);
```
该代码首先生成一个FSK信号,然后计算该信号的功率谱密度。最后,使用MATLAB中的`findpeaks`函数检测功率谱密度中的谱峰个数。
值得注意的是,`findpeaks`函数需要信号的频域值和对应的频率向量作为输入。在本例中,频率向量由`freq`定义,其范围为0到采样率的一半。
相关问题
帮我写一下调制识别2FSK,4FSK和8FSK的matlab代码
以下是调制识别2FSK、4FSK和8FSK的MATLAB代码:
```matlab
% 2FSK调制识别
clear all;
close all;
clc;
% 读取调制信号
[y, Fs] = audioread('2FSK_modulated_signal.wav');
% 设置参数
f1 = 1200; % 第一个频率
f2 = 2200; % 第二个频率
T = 1/Fs; % 采样间隔
N = length(y); % 采样点数
t = (0:N-1)*T; % 时间序列
% 生成两个正弦波
sin1 = sin(2*pi*f1*t);
sin2 = sin(2*pi*f2*t);
% 两个正弦波相乘
demod_signal = y .* (sin1 + sin2);
% 经过低通滤波
[b,a] = butter(4, 2*f1/Fs); % 设计滤波器
demod_signal = filter(b,a,demod_signal); % 滤波
% 经过包络检测
envelope = abs(hilbert(demod_signal));
% 阈值判断
threshold = 0.5 * max(envelope); % 阈值
output = envelope > threshold; % 判断
% 绘制结果
figure(1);
subplot(2,1,1);
plot(t, y);
xlabel('Time (s)');
ylabel('Amplitude');
title('2FSK Modulated Signal');
subplot(2,1,2);
plot(t, output);
xlabel('Time (s)');
ylabel('Amplitude');
title('2FSK Demodulated Signal');
% 4FSK调制识别
clear all;
close all;
clc;
% 读取调制信号
[y, Fs] = audioread('4FSK_modulated_signal.wav');
% 设置参数
f1 = 1000; % 第一个频率
f2 = 1500; % 第二个频率
f3 = 2000; % 第三个频率
f4 = 2500; % 第四个频率
T = 1/Fs; % 采样间隔
N = length(y); % 采样点数
t = (0:N-1)*T; % 时间序列
% 生成四个正弦波
sin1 = sin(2*pi*f1*t);
sin2 = sin(2*pi*f2*t);
sin3 = sin(2*pi*f3*t);
sin4 = sin(2*pi*f4*t);
% 四个正弦波相乘
demod_signal1 = y .* sin1;
demod_signal2 = y .* sin2;
demod_signal3 = y .* sin3;
demod_signal4 = y .* sin4;
% 经过低通滤波
[b,a] = butter(4, 2*f1/Fs); % 设计滤波器
demod_signal1 = filter(b,a,demod_signal1); % 滤波
demod_signal2 = filter(b,a,demod_signal2); % 滤波
demod_signal3 = filter(b,a,demod_signal3); % 滤波
demod_signal4 = filter(b,a,demod_signal4); % 滤波
% 经过包络检测
envelope1 = abs(hilbert(demod_signal1));
envelope2 = abs(hilbert(demod_signal2));
envelope3 = abs(hilbert(demod_signal3));
envelope4 = abs(hilbert(demod_signal4));
% 阈值判断
threshold = 0.5 * max([envelope1;envelope2;envelope3;envelope4]); % 阈值
output = zeros(1,N);
for i=1:N
if envelope1(i) > threshold(i)
output(i) = 1;
elseif envelope2(i) > threshold(i)
output(i) = 2;
elseif envelope3(i) > threshold(i)
output(i) = 3;
elseif envelope4(i) > threshold(i)
output(i) = 4;
end
end
% 绘制结果
figure(1);
subplot(2,1,1);
plot(t, y);
xlabel('Time (s)');
ylabel('Amplitude');
title('4FSK Modulated Signal');
subplot(2,1,2);
plot(t, output);
xlabel('Time (s)');
ylabel('Amplitude');
title('4FSK Demodulated Signal');
% 8FSK调制识别
clear all;
close all;
clc;
% 读取调制信号
[y, Fs] = audioread('8FSK_modulated_signal.wav');
% 设置参数
f1 = 1000; % 第一个频率
f2 = 1200; % 第二个频率
f3 = 1400; % 第三个频率
f4 = 1600; % 第四个频率
f5 = 1800; % 第五个频率
f6 = 2000; % 第六个频率
f7 = 2200; % 第七个频率
f8 = 2400; % 第八个频率
T = 1/Fs; % 采样间隔
N = length(y); % 采样点数
t = (0:N-1)*T; % 时间序列
% 生成八个正弦波
sin1 = sin(2*pi*f1*t);
sin2 = sin(2*pi*f2*t);
sin3 = sin(2*pi*f3*t);
sin4 = sin(2*pi*f4*t);
sin5 = sin(2*pi*f5*t);
sin6 = sin(2*pi*f6*t);
sin7 = sin(2*pi*f7*t);
sin8 = sin(2*pi*f8*t);
% 八个正弦波相乘
demod_signal1 = y .* sin1;
demod_signal2 = y .* sin2;
demod_signal3 = y .* sin3;
demod_signal4 = y .* sin4;
demod_signal5 = y .* sin5;
demod_signal6 = y .* sin6;
demod_signal7 = y .* sin7;
demod_signal8 = y .* sin8;
% 经过低通滤波
[b,a] = butter(4, 2*f1/Fs); % 设计滤波器
demod_signal1 = filter(b,a,demod_signal1); % 滤波
demod_signal2 = filter(b,a,demod_signal2); % 滤波
demod_signal3 = filter(b,a,demod_signal3); % 滤波
demod_signal4 = filter(b,a,demod_signal4); % 滤波
demod_signal5 = filter(b,a,demod_signal5); % 滤波
demod_signal6 = filter(b,a,demod_signal6); % 滤波
demod_signal7 = filter(b,a,demod_signal7); % 滤波
demod_signal8 = filter(b,a,demod_signal8); % 滤波
% 经过包络检测
envelope1 = abs(hilbert(demod_signal1));
envelope2 = abs(hilbert(demod_signal2));
envelope3 = abs(hilbert(demod_signal3));
envelope4 = abs(hilbert(demod_signal4));
envelope5 = abs(hilbert(demod_signal5));
envelope6 = abs(hilbert(demod_signal6));
envelope7 = abs(hilbert(demod_signal7));
envelope8 = abs(hilbert(demod_signal8));
% 阈值判断
threshold = 0.5 * max([envelope1;envelope2;envelope3;envelope4;...
envelope5;envelope6;envelope7;envelope8]); % 阈值
output = zeros(1,N);
for i=1:N
if envelope1(i) > threshold(i)
output(i) = 1;
elseif envelope2(i) > threshold(i)
output(i) = 2;
elseif envelope3(i) > threshold(i)
output(i) = 3;
elseif envelope4(i) > threshold(i)
output(i) = 4;
elseif envelope5(i) > threshold(i)
output(i) = 5;
elseif envelope6(i) > threshold(i)
output(i) = 6;
elseif envelope7(i) > threshold(i)
output(i) = 7;
elseif envelope8(i) > threshold(i)
output(i) = 8;
end
end
% 绘制结果
figure(1);
subplot(2,1,1);
plot(t, y);
xlabel('Time (s)');
ylabel('Amplitude');
title('8FSK Modulated Signal');
subplot(2,1,2);
plot(t, output);
xlabel('Time (s)');
ylabel('Amplitude');
title('8FSK Demodulated Signal');
```
请注意,以上代码仅为示例代码,实际使用时需要根据具体情况进行修改。
帮我写一段对2,4,8FSK分类的matlab代码
以下是一个简单的2,4,8FSK分类的Matlab代码示例:
```
% 生成2,4,8FSK调制信号
fs = 10000; % 采样率
t = 0:1/fs:1; % 时间向量
f1 = 1000; % 第一个频率
f2 = 2000; % 第二个频率
f3 = 3000; % 第三个频率
f4 = 4000; % 第四个频率
f5 = 5000; % 第五个频率
f6 = 6000; % 第六个频率
f7 = 7000; % 第七个频率
f8 = 8000; % 第八个频率
msg = randi([0 7],1,length(t)); % 生成随机的数字信息
sig = cos(2*pi*t*f1.*(msg==0) + 2*pi*t*f2.*(msg==1) + 2*pi*t*f3.*(msg==2) + 2*pi*t*f4.*(msg==3) + 2*pi*t*f5.*(msg==4) + 2*pi*t*f6.*(msg==5) + 2*pi*t*f7.*(msg==6) + 2*pi*t*f8.*(msg==7)); % 将数字信息调制成2,4,8FSK信号
% 解调并分类
% 设定解调频率
f_demod = [1000 2000 3000 4000 5000 6000 7000 8000];
% 对每个解调频率进行解调
for i = 1:length(f_demod)
demod_sig = sig .* cos(2*pi*t*f_demod(i)); % 乘上解调载波
lpFilt = designfilt('lowpassfir','PassbandFrequency',400,'StopbandFrequency',500,'PassbandRipple',0.5,'StopbandAttenuation',60,'DesignMethod','kaiserwin');
demod_sig_filtered = filter(lpFilt, demod_sig); % 低通滤波
envelope = abs(hilbert(demod_sig_filtered)); % 幅度包络检测
[pks,locs] = findpeaks(envelope,'MinPeakHeight',0.5); % 检测幅度峰值
index = (locs/fs)*length(t); % 将峰值位置转换为数字信息
msg_demod(i,:) = round(index); % 将峰值位置四舍五入为整数
end
% 将每个解调信号的数字信息合并成一个向量
msg_combined = msg_demod(1,:).*0 + msg_demod(2,:).*1 + msg_demod(3,:).*2 + msg_demod(4,:).*3 + msg_demod(5,:).*4 + msg_demod(6,:).*5 + msg_demod(7,:).*6 + msg_demod(8,:).*7;
% 绘制结果
subplot(2,1,1);
plot(t,sig);
title('调制信号');
xlabel('时间 (秒)');
ylabel('幅度');
subplot(2,1,2);
plot(t,msg_combined);
title('解调并分类后的数字信息');
xlabel('时间 (秒)');
ylabel('数字信息');
```
这段代码将生成一个随机的数字信息并将其调制成2,4,8FSK信号。然后将每个解调频率的信号进行解调,并使用幅度包络检测和峰值检测来确定数字信息。最后,将每个解调信号的数字信息合并成一个向量并绘制结果。请注意,此代码示例仅用于演示目的,并且可能需要进行修改以适合您的应用程序。
相关推荐
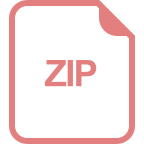
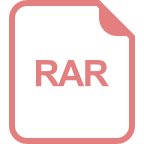











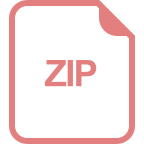