java图形化进程调度设计与实现_java实现模拟进程调度过程
时间: 2023-08-14 15:05:10 浏览: 89
Java图形化进程调度可以通过Java Swing等界面库实现。下面是一个简单的模拟进程调度过程的Java实现示例:
```java
import java.util.LinkedList;
import java.util.Queue;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class ProcessSchedulingSimulation extends JFrame {
private Queue<Process> readyQueue = new LinkedList<>();
private Process runningProcess = null;
private JLabel statusLabel;
public ProcessSchedulingSimulation() {
setTitle("Process Scheduling Simulation");
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel();
statusLabel = new JLabel("No process is running.");
panel.add(statusLabel);
add(panel);
setVisible(true);
}
private void addProcess(Process process) {
readyQueue.offer(process);
statusLabel.setText("Process " + process.getId() + " is added to the ready queue.");
if (runningProcess == null) {
runNextProcess();
}
}
private void runNextProcess() {
if (!readyQueue.isEmpty()) {
runningProcess = readyQueue.poll();
statusLabel.setText("Process " + runningProcess.getId() + " is running.");
runningProcess.run();
runningProcess = null;
runNextProcess();
} else {
statusLabel.setText("No process is running.");
}
}
public static void main(String[] args) {
ProcessSchedulingSimulation simulation = new ProcessSchedulingSimulation();
simulation.addProcess(new Process(1, 5));
simulation.addProcess(new Process(2, 3));
simulation.addProcess(new Process(3, 2));
simulation.addProcess(new Process(4, 6));
}
private static class Process {
private int id;
private int timeLeft;
public Process(int id, int time) {
this.id = id;
this.timeLeft = time;
}
public int getId() {
return id;
}
public void run() {
try {
Thread.sleep(timeLeft * 1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
在这个示例中,定义了一个`Process`类表示进程,包含进程ID和剩余执行时间。`ProcessSchedulingSimulation`类实现了一个简单的进程调度模拟,包含一个就绪队列`readyQueue`和一个正在运行的进程`runningProcess`。当新的进程加入就绪队列时,如果当前没有运行的进程,则立即运行队首的进程。运行过程中,进程会休眠对应的执行时间。当一个进程运行完毕后,调度器会尝试运行下一个进程,如果就绪队列为空,则当前没有进程正在运行。
在`main`方法中,创建了四个进程,并依次加入就绪队列,模拟了进程调度的过程。界面上的`statusLabel`会实时更新当前运行的进程信息。
相关推荐
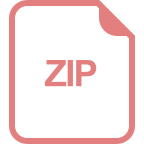
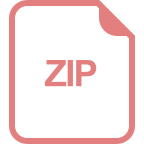











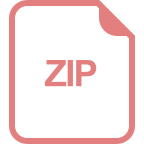
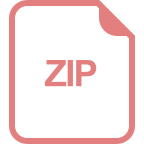
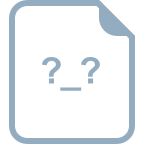
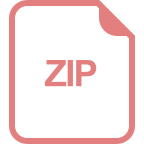