使用c语言队列的线程邮箱代码
时间: 2023-09-16 21:13:42 浏览: 89
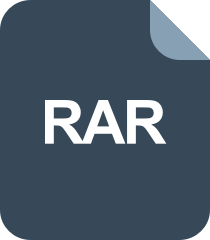
发邮件C语言代码

下面是一个使用C语言队列实现的线程邮箱代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define QUEUE_SIZE 10
// 队列结构体定义
typedef struct {
int data[QUEUE_SIZE];
int front;
int rear;
} Queue;
// 初始化队列
void initQueue(Queue *q) {
q->front = 0;
q->rear = 0;
}
// 判断队列是否已满
int isQueueFull(Queue *q) {
return ((q->rear + 1) % QUEUE_SIZE == q->front);
}
// 判断队列是否为空
int isQueueEmpty(Queue *q) {
return (q->front == q->rear);
}
// 入队
int enQueue(Queue *q, int data) {
if (isQueueFull(q)) {
return -1;
}
q->data[q->rear] = data;
q->rear = (q->rear + 1) % QUEUE_SIZE;
return 0;
}
// 出队
int deQueue(Queue *q, int *data) {
if (isQueueEmpty(q)) {
return -1;
}
*data = q->data[q->front];
q->front = (q->front + 1) % QUEUE_SIZE;
return 0;
}
// 生产者线程函数
void* producer(void *arg) {
Queue *q = (Queue*)arg;
int i;
for (i = 0; i < 20; i++) {
while (isQueueFull(q)) {
// 如果队列已满,等待
usleep(100000);
}
enQueue(q, i);
printf("Producer: %d\n", i);
}
return NULL;
}
// 消费者线程函数
void* consumer(void *arg) {
Queue *q = (Queue*)arg;
int data;
while (1) {
while (isQueueEmpty(q)) {
// 如果队列为空,等待
usleep(100000);
}
deQueue(q, &data);
printf("Consumer: %d\n", data);
}
return NULL;
}
int main() {
Queue q;
initQueue(&q);
pthread_t prod_tid, cons_tid;
pthread_create(&prod_tid, NULL, producer, &q);
pthread_create(&cons_tid, NULL, consumer, &q);
pthread_join(prod_tid, NULL);
pthread_join(cons_tid, NULL);
return 0;
}
```
在以上代码中,我们定义了一个队列结构体,并实现了队列的初始化、判断队列是否已满或为空、入队和出队等基本操作。在生产者线程函数中,我们不断向队列中写入数据,如果队列已满,则等待一段时间再重试;在消费者线程函数中,我们不断从队列中读取数据,如果队列已空,则等待一段时间再重试。
在主函数中,我们创建了一个生产者线程和一个消费者线程,并分别传入队列结构体的指针作为参数。最后,我们使用 `pthread_join` 函数等待两个子线程结束。
阅读全文
相关推荐
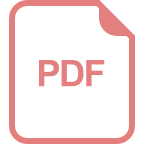



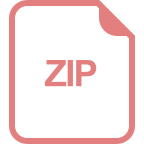
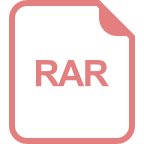
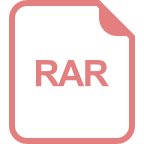
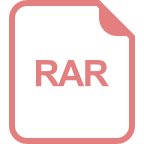
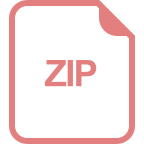
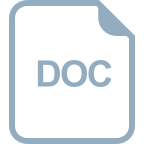
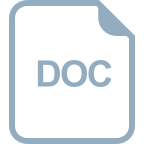
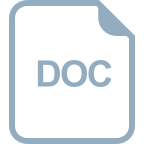
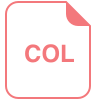
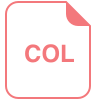

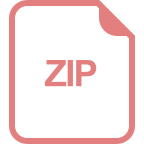