KeyError: 'category' 怎么解决这个报错
时间: 2024-01-17 21:03:50 浏览: 30
这个报错通常是因为你的代码中使用了一个字典或者 JSON 对象中不存在的键,你可以检查一下你的代码中是否有错误,或者检查一下字典或者 JSON 对象中是否真的包含了你所使用的键。如果确认一定存在这个键,你可以使用 try-except 语句来捕获 KeyError 异常并进行处理。例如:
```
try:
value = my_dict['category']
except KeyError:
value = None # 或者其他你希望的默认值
```
相关问题
keyerror: 0报错
这个错误通常表示你在尝试访问字典中不存在的键。请确保你正在访问正确的键,并且该键确实存在于字典中。你可以使用 `in` 关键字来检查字典中是否存在某个键,例如:
```python
my_dict = {"key1": "value1", "key2": "value2"}
if "key3" in my_dict:
print(my_dict["key3"]) # 这里不会报错,因为会先判断 "key3" 是否存在于 my_dict 中
else:
print("key3 不存在于 my_dict 中")
```
如果你确定要访问的键不存在于字典中,你可以使用 `get()` 方法来避免 `KeyError` 错误:
```python
my_dict = {"key1": "value1", "key2": "value2"}
print(my_dict.get("key3")) # 这里不会报错,因为 get() 方法会返回 None 而不是抛出 KeyError 错误
```
这段代码报错:KeyError: None
这个错误通常是由于尝试访问字典中不存在的键所引起的。在这段代码中,出现了一个 KeyError: None 的错误,这意味着代码尝试访问一个键为 None 的字典项,但该字典项不存在。
根据你提供的代码,我无法确定具体哪一行导致了此错误。请检查你的代码,看看是否有任何字典操作或索引操作,确保你正在使用正确的键。如果你需要更多的帮助,请提供更多的上下文或代码。
相关推荐
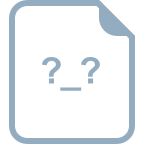
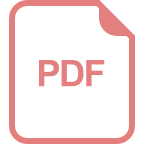
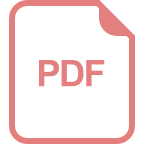












