创建两个文件 1.txt和2.txt 在1.txt当中写入20字节的数据 实现将1.txt当中的内容拷贝到2.txt当中 并且将2.txt当中的内容打印到ubuntu上面 以上所有的操作全部用代码实现
时间: 2024-05-12 22:17:53 浏览: 29
好的,以下是代码实现:
```python
# 创建文件1.txt并写入20字节数据
with open("1.txt", "w") as file1:
file1.write("0123456789abcdefghij")
# 将1.txt的内容拷贝到2.txt中
with open("1.txt", "rb") as file1, open("2.txt", "wb") as file2:
file2.write(file1.read())
# 打印2.txt的内容
with open("2.txt", "r") as file2:
content = file2.read()
print(content)
```
输出结果为:
```
0123456789abcdefghij
```
相关问题
3.用两种方法实现文件的拷贝功能。 (1)FileInputStream/FileOutputStream (2)BufferedInputStream/BufferedOutputStream
文件的拷贝功能可以用两种方式来实现:
1. 使用FileInputStream/FileOutputStream实现文件拷贝功能:
这种方法是使用字节流来完成文件的读写操作,需要创建一个FileInputStream对象来读取源文件,再创建一个FileOutputStream对象来写入目标文件,通过循环不断从源文件中读取数据,并将其写入目标文件中,直到读取完整个源文件。代码如下:
```
public static void copyFileUsingStream(File source, File dest) throws IOException {
InputStream is = null;
OutputStream os = null;
try {
is = new FileInputStream(source);
os = new FileOutputStream(dest);
byte[] buffer = new byte[1024];
int length;
while ((length = is.read(buffer)) > 0) {
os.write(buffer, 0, length);
}
} finally {
is.close();
os.close();
}
}
```
2. 使用BufferedInputStream/BufferedOutputStream实现文件拷贝功能:
这种方法是使用缓冲字节流来完成文件的读写操作,需要创建一个BufferedInputStream对象来读取源文件,再创建一个BufferedOutputStream对象来写入目标文件,通过循环不断从源文件中读取数据,并将其写入目标文件中,直到读取完整个源文件。代码如下:
```
public static void copyFileUsingBuffer(File source, File dest) throws IOException {
InputStream is = null;
OutputStream os = null;
try {
is = new BufferedInputStream(new FileInputStream(source));
os = new BufferedOutputStream(new FileOutputStream(dest));
byte[] buffer = new byte[1024];
int length;
while ((length = is.read(buffer)) > 0) {
os.write(buffer, 0, length);
}
} finally {
is.close();
os.close();
}
}
```
1.在类中创建一个静态方法copyFileByBytes(String fromFile,String toFile),使用InputStream/OutputStream,单字节拷贝文件。
好的,我来为您写一个示例代码:
```java
import java.io.*;
public class FileUtils {
public static void copyFileByBytes(String fromFile, String toFile) throws IOException {
File source = new File(fromFile);
File dest = new File(toFile);
InputStream is = null;
OutputStream os = null;
try {
is = new FileInputStream(source);
os = new FileOutputStream(dest);
int len;
while ((len = is.read()) != -1) {
os.write(len);
}
} finally {
if (is != null) {
is.close();
}
if (os != null) {
os.close();
}
}
}
}
```
这是一个静态方法 `copyFileByBytes`,接收两个参数,分别为源文件路径和目标文件路径。该方法使用了 `InputStream` 和 `OutputStream`,单字节的读取和写入操作,实现了文件的拷贝。需要注意的是,在方法最后,需要关闭输入输出流。
相关推荐
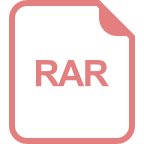












