vue3 $subscribe
时间: 2024-08-15 18:01:30 浏览: 46
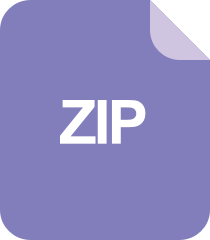
前端VUE3视频教程02
在 Vue 3 中,`$subscribe` 是Vuex 的一个核心概念,用于订阅状态(store state)的变化。Vuex 是一个专门为 Vue.js 应用程序设计的状态管理模式,它允许你在组件中集中管理共享数据状态。
`$subscribe` 方法是一个响应式的监听器,当你注册一个订阅时,它会在 store 的 state 发生更新时自动调用指定的方法。这样,你可以将对状态变化的处理逻辑解耦到单独的函数中,提高代码的可维护性和模块化。
使用 `$subscribe` 的基本语法通常是这样的:
```javascript
import { useStore } from 'vue3-store-module';
const store = useStore();
// 注册订阅
store.subscribe((mutation, state) => {
// 当 mutation 或者 state 更新时,会执行这个回调函数
console.log('State updated:', state);
});
// ...其他组件逻辑...
// 取消订阅
store.unsubscribe(subscriptionId); // 如果有提供订阅ID,否则直接传递匿名函数即可
```
相关问题:
1. `useStore()` 是从哪个库导入的?
2. 如何取消一个已注册的订阅?
3. 除了 `$subscribe`,Vue 3 的Vuex 还提供了哪些状态管理方法?
阅读全文
相关推荐
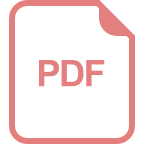
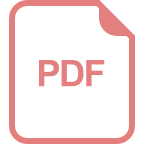















