通过js生成excel的blob文件后怎么修改excel单元格格式
时间: 2024-05-17 15:16:34 浏览: 109
生成Excel的blob文件后,需要借助第三方库来修改单元格格式。一种常用的库是js-xlsx。
具体步骤如下:
1. 解析blob文件,将其转为workbook对象:
```js
const reader = new FileReader();
reader.readAsArrayBuffer(blob);
reader.onload = () => {
const data = new Uint8Array(reader.result);
const workbook = XLSX.read(data, { type: "array" });
}
```
2. 获取worksheet对象:
```js
const sheetName = workbook.SheetNames[0];
const worksheet = workbook.Sheets[sheetName];
```
3. 设定单元格样式:
```js
const range = { s: { c: 0, r: 0 }, e: { c: 5, r: 0 } }; // 单元格范围
const cellStyle = { font: { bold: true }, alignment: { horizontal: "center" } }; // 单元格样式
worksheet["!cols"] = [{ width: 20 }, { width: 20 }, { width: 20 }, { width: 20 }, { width: 20 }, { width: 20 }]; // 设定列宽
worksheet["!merges"] = [{ ...range }]; // 合并单元格
for (let col = range.s.c; col <= range.e.c; col++) {
const cell = worksheet[XLSX.utils.encode_cell({ r: range.s.r, c: col })];
cell.s = cellStyle;
}
```
4. 生成新的blob文件:
```js
const newWorkbook = XLSX.utils.book_new();
XLSX.utils.book_append_sheet(newWorkbook, worksheet, sheetName);
const newBlob = new Blob([XLSX.write(newWorkbook, { type: "array", bookType: "xlsx" })]);
```
以上是一种修改Excel单元格格式的方法,具体操作还需根据实际需求进行调整。
阅读全文
相关推荐
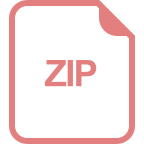
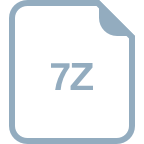
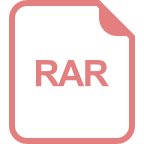
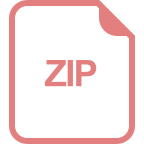
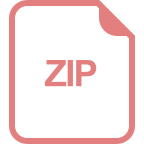
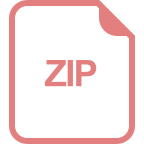
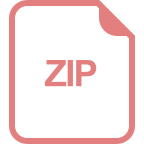
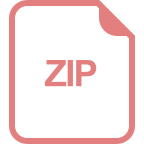
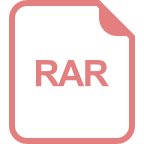
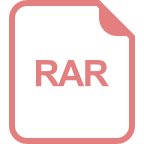
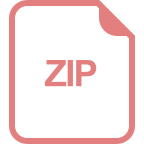
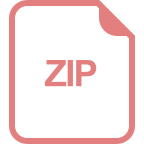
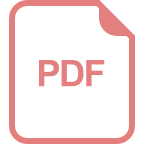
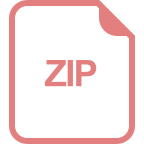
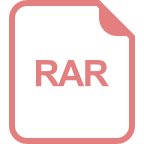
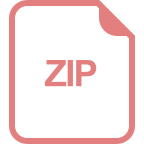
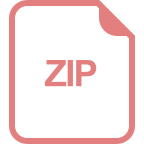
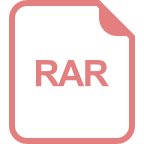